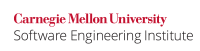
The conditional operator ?:
uses the boolean
value of one expression its first operand to decide which of the other two expressions should will be evaluated. (See JLS, Section 15 §15.25, "Conditional Operator ? :
" of the Java Language Specification (JLS) [JLS 2013].)
The general form of a Java conditional expression is operand1 ? operand2 : operand3
.
...
The conditional operator is syntactically right-associative; for . For example, a?b:c?d:e?f:g
is equivalent to a?b:(c?d:(e?f:g))
.
The JLS -defined rules for determining the result type of the result of a conditional expression (tabulated belowsee following table) are quite complicated; programmers could be surprised by the type conversions required for expressions they have written.
Result type determination begins from the top of the table; the compiler applies the first matching rule. The Operand 2 and Operand 3 columns refer to operand2
and operand3
(from the previous definition) respectively. In the table, constant int
refers to constant expressions of type int
(such as '0'
or variables declared final
) as constant int
; the "Operand 2" and "Operand 3" columns refer to operand2
and operand3
(from the above definition), respectively..
For the final table row, S1 and S2 are the types of the second and third operands respectively. T1 is the type that results from applying boxing conversion to S1, and T2 is the type that results from applying boxing conversion to S2. The type of the conditional expression is the result of applying capture conversion to S2. The type of the conditional expression is the result of applying capture conversion to the least upper bound of T1 and T2. See §5.1.7, "Boxing Conversion," §5.1.10, "Capture Conversion," and §15.12.2.7, "Inferring Type Arguments Based on Actual Arguments," of the JLS for additional information [JLS 2013].
Determining the Result Type of a Conditional Expression
Rule | Operand 2 | Operand 3 | Resultant |
---|
Type |
---|
1 |
Type T |
Type T |
Type T | |||
2 |
|
|
|
3 |
|
|
|
4 |
|
|
|
5 |
|
|
|
6 |
|
|
|
7 |
|
|
|
8 |
|
|
|
9 |
|
|
|
Byte
constant int
byte
if int
is representable as byte
constant int
Byte
byte
if int
is representable as byte
Short
constant int
short
if int
is representable as short
constant int
Short
short
if int
is representable as short
Character
constant int
char
if int
is representable as char
constant int
Character
char
if int
is representable as char
other numeric
other numeric
10 | Other numeric | Other numeric | Promoted type of the second and third operands |
11 | T1 = boxing conversion(S1) | T2 = boxing conversion(S2) |
Apply capture conversion to lub(T1,T2) |
See JLS, Section 5.1.7, "Boxing Conversion"; JLS, Section 5.1.10, "Capture Conversion"; and JLS, Section 15.12.2.7, "Inferring Type Arguments Based on Actual Arguments" for additional information on the final table entry.
The complexity of the rules that determine the result type of a conditional expression can lead to unintended type conversions. ThusConsequently, the second and third operands of each conditional expression should always have the same typeidentical types. This recommendation also applies to boxed primitives.
Noncompliant Code Example
This In this noncompliant code example prints , the programmer expects that both print statements will print the value of alpha
as A
, which is of the char
type. The third operand is a constant expression of type int
, whose value 0
can be represented as a char
; numeric promotion is unnecessary. However, this behavior depends on the particular value of the constant integer expression; changing that value can lead to different behavior. char
:
Code Block | ||
---|---|---|
| ||
public class Expr { public static void main(String[] args) { char alpha = 'A'; int i = 0; // Other code. Value of i may change boolean trueExp = true; // Some expression that evaluates to true System.out.print(true trueExp ? alpha : 0); // prints A System.out.print(trueExp ? alpha : 0i); // prints 65 } } |
The first print statement prints A
because the compiler applies rule 8 from the result type determination table to determine that the second and third operands of the conditional expression are, or are converted to, type char
. However, the second print statement prints 65
—the value of alpha
as an int
. The first matching rule from the table is rule 10. Consequently, the compiler promotes the value of alpha
to type int
.
Compliant Solution
This compliant solution uses identical types for the second and third operands of the each conditional expression; the explicit cast clarifies casts specify the expected type.type expected by the programmer:
Code Block | ||
---|---|---|
| ||
public class Expr { public static void main(String[] args) { char alpha = 'A'; // Castint i = 0; as a char toboolean explicitlytrueExp state that the type of the = true; // conditionalExpression expressionthat shouldevaluates beto char.true System.out.print(truetrueExp ? alpha : ((char) 0)); // } } |
Noncompliant Code Example
This noncompliant example prints {{65}}â”the ASCII equivalent of A
, instead of the expected A
, because the second operand (alpha
) must be promoted to type int
. The numeric promotion occurs because the value of the third operand (the constant expression '12345') is too large to be represented as a char
.
Code Block | ||
---|---|---|
| ||
public class Expr { public static void main(String[] args) { char alpha = 'A';Prints A // Deliberate narrowing cast of i; possible truncation OK System.out.print(true trueExp ? alpha : : 12345);((char) i)); // Prints A } } |
Compliant Solution
The compliant solution explicitly states the intended result type by casting alpha
to type int
. Casting 12345
to type char
would ensure that both operands of the conditional expression have the same type, resulting in A
being printed. However, it would result in data loss when an integer larger than Character.MAX_VALUE
is downcast to type char
. This compliant solution avoids potential truncation by casting alpha
to type int
, the wider of the operand types.
When the value of i
in the second conditional expression falls outside the range that can be represented as a char
, the explicit cast will truncate its value. This usage complies with exception NUM12-J-EX0 of NUM12-J. Ensure conversions of numeric types to narrower types do not result in lost or misinterpreted data.
Noncompliant Code Example
This noncompliant code example prints 100 as the size of the HashSet
rather than the expected result (some value between 0 and 50):
Code Block | ||
---|---|---|
| ||
public class ShortSet | ||
Code Block | ||
| ||
public class Expr { public static void main(String[] args) { charHashSet<Short> alphas = 'A'new HashSet<Short>(); for //(short Casti alpha= as0; ani int< to explicitly state that the type of the 100; i++) { s.add(i); // conditional expression should be int. Cast of i-1 is safe because value is always representable System.out.print(true ? ((int) alpha) : 12345); } } |
Noncompliant Code Example
This noncompliant code example prints 65
instead of A
. The third operand is a variable of type int
, so the second operand (alpha
) must be converted to type int
.
Code Block | ||
---|---|---|
| ||
public class Expr { public static void main(String[] args) { char alpha = 'A'; int i = 0;Short workingVal = (short) (i-1); // ... Other code may update workingVal s.remove(((i % 2) == 1) ? i-1 : workingVal); } System.out.println(s.print(true ? alpha : isize()); } } |
Compliant Solution
This compliant solution declares i
as type char
, ensuring that the second and third operands of the conditional expression have the same type.
The combination of values of types short
and int
in the second argument of the conditional expression (the operation i-1
) causes the result to be an int
, as specified by the integer promotion rules. Consequently, the Short
object in the third argument is unboxed into a short
, which is then promoted into an int
. The result of the conditional expression is then autoboxed into an object of type Integer
. Because the HashSet
contains only values of type Short
, the call to HashSet.remove()
has no effect.
Compliant Solution
This compliant solution casts the second operand to type short
, then explicitly invokes the Short.valueOf()
method to create a Short
instance whose value is i-1
:
Code Block | ||
---|---|---|
| ||
public class ExprShortSet { public static void main(String[] args) { charHashSet<Short> alphas = 'A' new HashSet<Short>(); charfor (short i = 0; //declarei as< char System.out.print(true ? alpha : 100; i++) { s.add(i); } } |
Noncompliant Code Example
...
|
...
|
...
|
...
|
...
// Cast of |
...
i-1 |
...
is |
...
Code Block | ||
---|---|---|
| ||
public class Expr {
public static void main(String[] args) {
Integer i = Integer.MAX_VALUE;
float f = 0;
System.out.print(true ? i : f);
}
}
|
Compliant Solution
This compliant solution declares both the operands as Integer
.
Code Block | ||
---|---|---|
| ||
public class Expr { public static void main(String[] args) { Integer i = Integer.MAX_VALUE; Integer f = 0; //declare as Integersafe because the resulting value is always representable Short workingVal = (short) (i-1); // ... Other code may update workingVal // Cast of i-1 is safe because the resulting value is always representable s.remove(((i % 2) == 1) ? Short.valueOf((short) (i-1)) : workingVal); } System.out.print(true ? i : fprintln(s.size()); } } |
...
As a result of the cast, the second and third operands of the conditional expression both have type Short
, and the remove()
call has the expected result.
Writing the conditional expression as ((i % 2) == 1) ? (short) (i-1)) : workingVal
also complies with this guideline because both the second and third operands in this form have type short
. However, this alternative is less efficient because it forces unboxing of workingVal
on each even iteration of the loop and autoboxing of the result of the conditional expression (from short
to Short
) on every iteration of the loop.
Applicability
When the second and third operands of a conditional expression have different types, they can be subject to unexpected type conversions that were not anticipated by the programmer.
...
Guideline
...
Severity
...
Likelihood
...
Remediation Cost
...
Priority
...
Level
...
...
low
...
unlikely
...
medium
...
P2
...
L3
Automated Detection
Automated detection of condition expressions whose second and third operands are of different types is straightforward.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
Wiki Markup |
---|
\[[Bloch 2005|AA. Bibliography#Bloch 05]\] Puzzle 8: Dos Equis
\[[Findbugs 2008|AA. Bibliography#Findbugs 08]\] "Bx: Primitive value is unboxed and coerced for ternary operator"
\[[JLS 2005|AA. Bibliography#JLS 05]\] [Section 15.25|http://java.sun.com/docs/books/jls/third_edition/html/expressions.html#15.25], "Conditional Operator {{? :}}" |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Parasoft Jtest |
| CERT.EXP55.COMT | Avoid using the conditional operator with mismatched numeric types |
Bibliography
Puzzle 8, "Dos Equis" | |
"Bx: Primitive Value Is Unboxed and Coerced for Ternary Operator" | |
[JLS 2013] | §15.25, "Conditional Operator ? : " |
...
EXP13-J. Consistently use the symbolic constants you define 04. Expressions (EXP) 05. Scope (SCP)