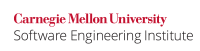
A switch
statement consists of several case labellabels, plus a default label. The default label is optional , but recommended. (see See MSC01-C. Strive for logical completeness.) . A series of statements following a case label conventionally end ends with a break;
statement; if omitted, control flow falls through to the next case in the switch
statement block. Since Because the break
statement is not required, omitting the break statement it does not produce compiler warnings, and thus can produce diagnostics. If the omission was unintentional, it can result in an unexpected control flow.
Noncompliant Code Example
In this noncompliant code example, the case for when where widget_type
is WE_W
lacks a break
statement. Consequently, the statementsfor statements that should be executed only when widget_type
is WE_X
get are executed even when widget_type
is WE_W
.
Code Block | ||||
---|---|---|---|---|
| ||||
enum WidgetEnum { WE_W, WE_X, WE_Y, WE_Z } widget_type; widget_type = WE_X; switch (widget_type) { case WE_W: /* ... */ case WE_X: /* ... */ break; case WE_Y: case WE_Z: /* ... */ break; default: /* canCan't happen */ /* handleHandle error condition */ } |
Compliant Solution
In this compliant solution, each sequence of statements following a case label ends with a break
statement:
Code Block | ||||
---|---|---|---|---|
| ||||
enum WidgetEnum { WE_W, WE_X, WE_Y, WE_Z } widget_type; widget_type = WE_X; switch (widget_type) { case WE_W: /* ... */ break; case WE_X: /* ... */ break; case WE_Y: case WE_Z: /* ... */ break; default: /* canCan't happen */ /* handleHandle error condition */ } |
A break
statement is not required following the case where widget_type
is WE_Y
because there are no statements before the next case label, indicating that both WE_Y
and WE_Z
should be handled in the same fashion.
A break
statement is not required following the default case because it would not affect the control flow.
Exceptions
MSC17:-C-EX1: The last label in a switch
statement requires no final break
. This It will conventionally be the default
label.
MSC17:-C-EX2: When control flow is intended to cross statement labels, it is permissible to omit the break
statement. In these instances, the unusual control flow must be explicitly documentedmade clear, such as by adding the [[fallthrough]]
C2x attribute, the __attribute__((__fallthrough__))
GNU extension, or a simple comment.
Code Block | ||||
---|---|---|---|---|
| ||||
enum WidgetEnum { WE_W, WE_X, WE_Y, WE_Z } widget_type; widget_type = WE_X; switch (widget_type) { case WE_W: /* ... */ /* noNo break,; fall processthrough caseto forthe WE_X as wellcase */ case WE_X: /* ... */ break; case WE_Y: case WE_Z: /* ... */ break; default: /* canCan't happen */ /* handleHandle error condition */ } |
Risk Assessment
Failure to include break
statements leads to unexpected control flow.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MSC17-C |
Medium |
Likely |
Low |
P18 |
L1 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Astrée |
| switch-clause-break switch-clause-break-continue switch-clause-break-return | Fully checked | ||||||
CodeSonar |
|
Compass/ROSE can detect violations of this recommendation.
LANG.STRUCT.SW.MB | Missing break | ||||||||
Compass/ROSE | |||||||||
| MISSING_BREAK | Can find instances of missing break statement between cases in | |||||||
| CC2.MSC17 | Fully implemented | |||||||
Helix QAC |
| C2003 | |||||||
Klocwork |
| MISRA.SWITCH.WELL_FORMED.BREAK.2012 | |||||||
LDRA tool suite |
| 62 S | Fully implemented | ||||||
Parasoft C/C++test |
| CERT_C-MSC17-a | Missing break statement between cases in a switch statement | ||||||
PC-lint Plus |
| 616, 825 | Fully supported | ||||||
| CERT C: Rec. MSC17-C | Checks for missing break of switch case (rec. fully covered) | |||||||
PVS-Studio |
| V796 | |||||||
RuleChecker |
| switch-clause-break switch-clause-break-continue switch-clause-break-return | Fully checked | ||||||
SonarQube C/C++ Plugin |
| NonEmptyCaseWithoutBreak |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
...
Related Guidelines
...
...
...
CERT Oracle Secure Coding Standard for Java | MSC52-J. Finish every set of statements associated with a case label with a break statement |
...
References
MSC16-C. Consider encrypting function pointers 49. Miscellaneous (MSC)