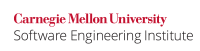
The C99 standard \[The C Standard, 6.7.3.2, paragraph 20 [ISO/IEC 9899:1999|AA. C References#ISO/IEC 9899-1999]\] introduces flexible array members into the language. While flexible array members are a useful addition they need to be understood and used with care. 2024], says Wiki Markup
As a special case, the last element of a structure with more than one named member may have an incomplete array type; this is called a flexible array member. In most situations, the flexible array member is ignored. In particular, the size of the structure is as if the flexible array member were omitted except that it may have more trailing padding than the omission would imply.
The following is an example of a structure that contains a flexible array member:
Code Block |
---|
struct flexArrayStructflex_array_struct { int num; int data[]; }; |
This definition means that , when allocating storagewhen computing the size of such a structure, only the first member, num
, is considered. ConsequentlyUnless the appropriate size of the flexible array member has been explicitly added when allocating storage for an object of the struct
, the result of accessing the member data
of a variable of type struct flexArrayStruct
nonpointer type struct flex_array_struct
is undefined. DCL38-C. Use the correct syntax when declaring a flexible array membersmember describes the correct way to declare a struct
with a flexible array member.
To avoid the potential for undefined behavior, structures that contain a flexible array member should always be allocated dynamically. Flexible array structures must
- Have dynamic storage duration (be allocated
...
- via
malloc()
or another dynamic allocation function) - Be dynamically copied using
memcpy()
or a similar function and not by assignment - When used as an argument to a function, be passed by pointer and not copied by value
Noncompliant Code Example (Storage
...
Duration)
This noncompliant code example statically allocates uses automatic storage for a structure containing a flexible array member.:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stddef.h> struct flexArrayStruct flexStruct; flex_array_struct { size_t array_size; size_t i; /* Initializenum; int data[]; }; void func(void) { struct flex_array_struct flex_struct; size_t array_size */= 4; /* Initialize structure */ flexStruct flex_struct.num = 0array_size; for (size_t i = 0; i < array_size; i++i) { flexStruct flex_struct.data[i] = 0; } |
Wiki Markup |
---|
The problem with this code is that the {{flexArrayStruct}} does not actually reserve space for the integer array data - it can't as the size hasn't been specified. Consequently, while initializing the {{num}} member to zero is allowed, attempting to write even one value into data (that is, {{data\[0\]}}) is likely to overwrite memory outside of the bounds of the object. |
} |
Because the memory for flex_struct
is reserved on the stack, no space is reserved for the data
member. Accessing the data
member is undefined behavior.
Compliant Solution (Storage Duration
...
)
This compliant solution dynamically allocates storage for struct flexArrayStruct
. flex_array_struct
:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> struct flexArrayStruct *flexStruct; flex_array_struct { size_t array_sizenum; size_t i int data[]; /* Initialize}; void func(void) { struct flex_array_struct *flex_struct; size_t array_size = */4; /* Dynamically allocate memory for the structurestruct */ flexStruct flex_struct = (struct flexArrayStructflex_array_struct *)malloc( sizeof(struct flexArrayStruct) flex_array_struct) + sizeof(int) * array_size ); if (flexStructflex_struct == NULL) { /* Handle mallocerror failure */ } /* Initialize structure */ flexStruct flex_struct->num = 0array_size; for (size_t i = 0; i < array_size; i++i) { flexStruct flex_struct->data[i] = 0; } |
...
|
...
|
...
} |
...
} |
Noncompliant Code Example (Copying)
When using structures with a flexible array member you should never directly copy an instance of the structure. This noncompliant code example attempts to replicate a copy an instance of struct flexArrayStruct
.a structure containing a flexible array member (struct
) by assignment:flex_array_struct
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stddef.h> struct flexArrayStruct *flexStructA; struct flexArrayStruct *flexStructB; flex_array_struct { size_t array_sizenum; size_t i int data[]; }; /* Initialize void func(struct flex_array_sizestruct */ /* Allocate memory for flexStructA */ /* Allocate memory for flexStructB */ /* Initialize flexStructA */ /* ... */ *flexStructB = *flexStructA; struct_a, struct flex_array_struct *struct_b) { *struct_b = *struct_a; } |
When the structure is copied, The problem with this noncompliant code example is that when the structure is copied the size of the flexible array member is not considered, and only the first member of the structure, num
, is copied, leaving the array contents untouched.
Compliant Solution (Copying)
This compliant solution uses memcpy()
to properly copy the content of flexStructA
into flexStructB
.of struct_a
into struct_b
:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <string.h> struct flexArrayStruct *flexStructA; struct flexArrayStruct *flexStructB; flex_array_struct { size_t array_sizenum; size_t i int data[]; }; /* Initialize void func(struct flex_array_sizestruct */ /* Allocate memory for flexStructA */ /* Allocate memory for flexStructB */ /* Initialize flexStructA */ /* ... */ memcpy(flexStructB, flexStructA, (sizeof(struct flexArrayStruct) + sizeof(int) * array_size)); |
...
struct_a,
struct flex_array_struct *struct_b) {
if (struct_a->num > struct_b->num) {
/* Insufficient space; handle error */
return;
}
memcpy(struct_b, struct_a,
sizeof(struct flex_array_struct) + (sizeof(int)
* struct_a->num));
} |
Noncompliant Code Example (Function Arguments)
When using structures with a flexible array member you should never directly pass an instance of the structure in a function call. In this noncompliant code example, the flexible array structure is passed directly by value to a function which tries to print that prints the array elements.:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <stdlib.h> struct flex_array_struct void print_array(struct flexArrayStruct structP) { size_t i num; int data[]; }; void printfprint_array(struct flex_array_struct struct_p) { puts("Array is: "); for (size_t i = 0; i < structPstruct_p.num; i++i) { printf("%d ", structPstruct_p.data[i]); } printfputchar("'\n"'); } void func(void) { struct flexArrayStructflex_array_struct *structPstruct_p; size_t array_size; size_t i = 4; /* initialize array_size */ /* spaceSpace is allocated for the struct */ structP struct_p = (struct flexArrayStructflex_array_struct *)malloc( sizeof(struct flexArrayStructflex_array_struct) + sizeof(int) * array_size ); if (structPstruct_p == NULL) { /* Handle malloc failureerror */ } structP struct_p->num = array_size; for (size_t i = 0; i < array_size; i++i) { structP struct_p->data[i] = i; } print_array(*structPstruct_p); } |
Because the argument is passed by value, the size of the flexible array member is not considered when the structure is copied, and only the first member of the structure, num
, is copiedThe problem with this code is that passing the structure directly to the function actually makes a copy of the structure. This copied fails for the same reason as the copy example above.
Compliant Solution (Function Arguments)
Never allow a structure with a flexible array member to be passed directly in a function call. The above code can be fixed by changing the function to accept a pointer to the structure.In this compliant solution, the structure is passed by reference and not by value:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <stdlib.h> struct flex_array_struct void print_array(struct flexArrayStruct *structP) { size_t i num; int data[]; }; void printfprint_array(struct flex_array_struct *struct_p) { puts("Array is: "); for (size_t i = 0; i < structPstruct_p->num; i++i) { printf("%d ", structPstruct_p->data[i]); } putsputchar("'\n"'); } void func(void) { struct flexArrayStructflex_array_struct *structPstruct_p; size_t array_size; size_t i = 4; /* initialize array_size */ /* spaceSpace is allocated for the struct */ structP = (struct flexArrayStruct *)malloc( sizeof(struct flexArrayStruct) + sizeof(int) * array_size ); if (structP == NULL) { /* Handle malloc failure */ } structP->num = array_size; for (i = 0; i < array_size; i++) { structP->data[i] = i; } print_array(structP); and initialized... */ print_array(struct_p); } |
Risk Assessment
Failure to use structures with flexible array members correctly can result in undefined behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MEM33-C |
Low |
Unlikely |
Low | P3 | L3 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Astrée |
| flexible-array-member-assignment flexible-array-member-declaration | Fully checked | ||||||
Axivion Bauhaus Suite |
| CertC-MEM33 | Fully implemented | ||||||
CodeSonar |
| LANG.STRUCT.DECL.FAM | Declaration of Flexible Array Member | ||||||
Compass/ROSE |
...
Can detect |
...
all of these | |||||||||
Cppcheck Premium |
| premium-cert-mem33-c | Partially implemented | ||||||
Helix QAC |
| C1061, C1062, C1063, C1064 | |||||||
Klocwork |
| MISRA.INCOMPLETE.STRUCT | |||||||
LDRA tool suite |
| 649 S, 650 S | Fully implemented | ||||||
Parasoft C/C++test |
| CERT_C-MEM33-a | Allocate structures containing a flexible array member dynamically | ||||||
| CERT C: Rule MEM33-C | Checks for misuse of structure with flexible array member (rule fully covered) | |||||||
RuleChecker |
| flexible-array-member-assignment flexible-array-member-declaration | Fully checked |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
Key here (explains table format and definitions)
Taxonomy | Taxonomy item | Relationship |
---|---|---|
CERT C Secure Coding Standard | DCL38-C. |
...
Use the correct syntax when declaring a flexible array member | Prior to 2018-01-12: CERT: Unspecified Relationship |
CERT-CWE Mapping Notes
Key here for mapping notes
CWE-401 and MEM33-CPP
There is no longer a C++ rule for MEM33-CPP. (In fact, all C++ rules from 30-50 are gone, because we changed the numbering system to be 50-99 for C++ rules.)
Bibliography
References
...
[ISO/IEC 9899:2024] | Subclause 6.7.3.2, "Structure and Union Specifiers" |
...
N791] | Solving the Struct Hack Problem |
...
|http://www.open-std.org/jtc1/sc22/wg14/www/docs/n791.htm]\]MEM32-C. Detect and handle memory allocation errors 08. Memory Management (MEM)