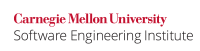
Bit-fields can be used to allow flags or other integer values with small ranges to be packed together to save storage space.
It is implementation-defined whether the specifier int
designates the same type as signed int
or the same type as unsigned int
for bit-fields. According to C99 to the C Standard [ISO/IEC 9899:19992011], C integer promotions also require that "if an int
can represent all values of the original type (as restricted by the width, for a bit-field), the value is converted to an int
; otherwise, it is converted to an unsigned int
."
This is a similar issue This issue is similar to the signedness of plain char
, discussed in recommendation INT07-C. Use only explicitly signed or unsigned char type for numeric values. A plain int
bit-field that is treated as unsigned will promote to int
, as long as its field width is less than that of int
because int
can hold all values of the original type. This behavior is the same behavior as that of a plain char
treated as unsigned. However, a plain int
bit-field treated as unsigned will promote to unsigned int
, if its field width is the same as that of int
. This difference makes a plain int
bit-field even trickier than a plain char
.
Bit-field types other than _Bool
, int
, signed int
, and unsigned int
are implementation-defined. They still obey the integer promotions quoted above previously when the specified width is at least as narrow as CHAR_BIT*sizeof(int)
, but wider bit-fields are not portable.
...
This noncompliant code depends on implementation-defined behavior. It prints either -1
or 255
, depending on whether a plain int
bit-field is signed or unsigned.
Code Block | ||||
---|---|---|---|---|
| ||||
struct {
int a: 8;
} bits = {255};
int main(void) {
printf("bits.a = %d.\n", bits.a);
return 0;
}
|
...
This compliant solution uses an unsigned int
bit-field and does not depend on implementation-defined behavior.:
Code Block | ||||
---|---|---|---|---|
| ||||
struct {
unsigned int a: 8;
} bits = {255};
int main(void) {
printf("bits.a = %d.\n", bits.a);
return 0;
}
|
...
Making invalid assumptions about the type of a bit-field or its layout can result in unexpected program flow.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
INT12-C |
Low |
Unlikely |
Medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|
Astrée |
| bitfield-type | Fully checked | ||||||
Axivion Bauhaus Suite |
| CertC-INT12 | |||||||
CodeSonar |
| LANG.TYPE.BFSIGN | Bit-field signedness not explicit | ||||||
Compass/ROSE | |||||||||
| CC2.INT12 | Fully implemented | |||||||
Helix QAC |
| C0634, C0635 | |||||||
Klocwork |
| MISRA.BITFIELD.TYPE | |||||||
LDRA tool suite |
|
|
|
73 S |
Section |
---|
Fully Implemented |
Section |
---|
Compass/ROSE |
Fully implemented | |||||||||
Parasoft C/C++test |
| CERT_C-INT12-a | Bit fields shall only be defined to be of type unsigned int or signed int | ||||||
PC-lint Plus |
| 846 | Fully supported | ||||||
Polyspace Bug Finder |
| Checks for bit-field declared without appropriate type (rec. fully covered) | |||||||
RuleChecker |
| bitfield-type | Fully checked | ||||||
SonarQube C/C++ Plugin |
| S814 |
Section |
---|
Section |
---|
bitftype |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
...
...
VOID INT12-CPP. Do not make assumptions about the type of a plain int bit-field when used in an expression | |
ISO/IEC |
...
TR 24772:2013 | Bit Representations [STR] |
MISRA C:2012 | Rule 10.1 (required) |
Bibliography
[ISO/IEC 9899:2011] | Subclause 6.3.1.1, "Boolean, Characters, and Integers" |
...
ISO/IEC TR 24772 "STR Bit Representations"
MISRA Rule 12.7
Bibliography
INT11-C. Take care when converting from pointer to integer or integer to pointer 04. Integers (INT)