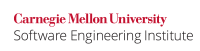
...
In C, function arguments are passed by value rather than by reference. Although a function may change the values passed in, these changed values are discarded once the function returns. For this reason, many programmers assume a function will not change its arguments , and that declaring the function's parameters as const
is unnecessary.
Code Block |
---|
void foo(int x) { x = 3; /* persistsVisible only untilin the function exits */ /* ... */ } |
Pointers behave in a similar fashion. A function may change a pointer to reference a different object, or NULL
, yet that change is discarded once the function exits. Consequently, declaring a pointer as const
is unnecessary.
Code Block |
---|
void foo(int *x) { x = NULL; /* persistsVisible only untilin the function exits */ /* ... */ } |
Noncompliant Code Example
Unlike passed-by-value arguments and pointers, pointed-to values are a concern. A function may modify a value referenced by a pointer argument, leading to a side effect that persists even after the function exits. Modification of the pointed-to value is not diagnosed by the compiler, which assumes this behavior was the intended behavior.
Code Block | ||||
---|---|---|---|---|
| ||||
void foo(int *x) { if (x != NULL) { *x = 3; /* visibleVisible outside function */ } /* ... */ } |
If the function parameter is const
-qualified, any attempt to modify the pointed-to value results in a fatal diagnosticshould cause the compiler to issue a diagnostic message.
Code Block | ||||
---|---|---|---|---|
| ||||
void foo(const int *x) { if (x != NULL) { *x = 3; /* Compiler should generatesgenerate compilerdiagnostic errormessage */ } /* ... */ } |
As a result, the const
violation must be resolved before the code can be compiled without a diagnostic message being issued.
Compliant Solution
This compliant solution addresses the const
violation by not modifying the constant argument.:
Code Block | ||||
---|---|---|---|---|
| ||||
void foo(const int * x) { if (x != NULL) { printf("Value is %d\n", *x); } /* ... */ } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
char *strcat_nc(char *s1, char *s2); char *c_str1 = "c_str1"; const char *c_str2 = "c_str2"; char c_str3[9] = "c_str3"; const char c_str4[9] = "c_str4"; strcat_nc(c_str3, c_str2); /* Compiler warns that c_str2 is const */ strcat_nc(c_str1, c_str3); /* Attempts to overwrite string literal! */ strcat_nc(c_str4, c_str3); /* Compiler warns that c_str4 is const */ |
The function behaves the same as strcat()
, but the compiler generates warnings in incorrect locations and fails to generate them in correct locations.
In the first strcat_nc()
call, the compiler generates a warning about attempting to cast away const
on c_str2
. This is because strcat_nc()
does not modify its second argument , yet fails to declare it const
.
In the second strcat_nc()
call, the compiler compiles the code with no warnings, but the resulting code will attempt to modify the "c_str1"
literal. This violates STR05-C. Use pointers to const when referring to string literals and STR30-C. Do not attempt to modify string literals.
In the final strcat_nc()
call, the compiler generates a warning about attempting to cast away const
on c_str4
. This , which is a valid warning.
Compliant Solution
...
Code Block | ||||
---|---|---|---|---|
| ||||
char *strcat(char *s1, const char *s2); char *c_str1 = "c_str1"; const char *c_str2 = "c_str2"; char c_str3[9] = "c_str3"; const char c_str4[9] = "c_str4"; strcat(c_str3, c_str2); /* Args reversed to prevent overwriting string literal */ strcat(c_str3, c_str1); strcat(c_str4, c_str3); /* Compiler warns that c_str4 is const */ |
The const
-qualification of the second argument, s2
, eliminates the spurious warning in the initial invocation but maintains the valid warning on the final invocation in which a const
-qualified object is passed as the first argument (which can change). Finally, the middle strcat()
invocation is now valid , as because c_str3
is a valid destination string and may be safely modified.
Risk Assessment
Not declaring Failing to declare an unchanging value const
prohibits the function from working with values already cast as const
. This problem can be sidestepped by type casting away the const
, but doing so violates EXP05-C. Do not cast away a const qualification.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
DCL13-C |
Low |
Unlikely |
Low | P3 | L3 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Astrée |
| parameter-missing-const | Fully checked | ||||||
Axivion Bauhaus Suite |
| CertC-DCL13 | |||||||
CodeSonar |
| LANG.TYPE.CBCONST | Pointed-to Type Could Be const | ||||||
Compass/ROSE |
Can detect violations of this recommendation while checking for violations of recommendation DCL00-C. Const-qualify immutable objects |
|
CC2.DCL13 | Fully implemented | ||||||||
Helix QAC |
| C0431, C3673, C3677 | |||||||
Klocwork |
| MISRA.PPARAM.NEEDS.CONST | |||||||
LDRA tool suite |
|
120 D | Fully implemented |
3673
0431(C)
Parasoft C/C++test |
| CERT_C-DCL13-a | A pointer parameter in a function prototype should be declared as pointer to const if the pointer is not used to modify the addressed object | ||||||
PC-lint Plus |
| 111, 818 | Fully supported | ||||||
Polyspace Bug Finder |
| CERT C: Rec. DCL13-C | Checks for pointer to non-const qualified function parameter (rec. fully covered) | ||||||
RuleChecker |
| parameter-missing-const | Fully checked |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
SEI CERT C++ |
Coding Standard | VOID DCL13-CPP. Declare function parameters that are pointers to values not changed by the function as const |
ISO/IEC TR 24772:2013 | Passing |
Parameters and |
Return Values [CSJ] |
...
MISRA C:2012 | Rule 8.13 (advisory) |
...