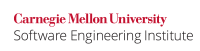
...
Methods
...
can
...
return
...
values
...
to
...
communicate
...
failure
...
or
...
success
...
or
...
to
...
update
...
local
...
objects
...
or
...
fields.
...
Security
...
risks
...
can
...
arise
...
when
...
method
...
return
...
values
...
are
...
ignored
...
or
...
when
...
the
...
invoking
...
method
...
fails
...
to
...
take
...
suitable
...
action.
...
Consequently,
...
programs
...
must
...
not
...
ignore
...
method
...
return
...
values.
...
When
...
getter
...
methods
...
are
...
named
...
after
...
an
...
action,
...
a
...
programmer
...
could
...
fail
...
to
...
realize
...
that
...
a
...
return
...
value
...
is
...
expected.
...
For
...
example,
...
the
...
only
...
purpose
...
of
...
the
...
ProcessBuilder.redirectErrorStream()
...
method
...
is
...
to
...
report
...
via
...
return
...
value
...
whether
...
the
...
process
...
builder
...
successfully
...
merged standard
...
error
...
and
...
standard
...
output.
...
The
...
method
...
that
...
actually
...
performs
...
redirection
...
of
...
the
...
error
...
stream
...
is
...
the
...
overloaded
...
single-argument method ProcessBuilder.redirectErrorStream(boolean)
.
Noncompliant Code Example (File Deletion)
This noncompliant code example attempts to delete a file but fails to check whether the operation has succeeded:
Code Block | ||
---|---|---|
| ||
version. {mc} Another example is ignoring the return value from add() on a HashSet. If duplicate, false will be returned. {mc} h2. Noncompliant Code Example (File Deletion) This noncompliant code example attempts to delete a file but fails to check whether the operation has succeeded. {code:bgColor=#FFcccc} public void deleteFile(){ File someFile = new File("someFileName.txt"); // doDo something with someFile someFile.delete(); } {code} h2. Compliant Solution This compliant solution checks the ({{boolean}}) value returned by the {{delete()}} method and handles any resulting errors. {code:bgColor=#ccccff} |
Compliant Solution
This compliant solution checks the Boolean value returned by the delete()
method and handles any resulting errors:
Code Block | ||
---|---|---|
| ||
public void deleteFile(){ File someFile = new File("someFileName.txt"); // doDo something with someFile if (!someFile.delete()) { // handleHandle failure to delete the file } } {code} h2. Noncompliant Code Example |
Noncompliant Code Example (String
...
Replacement)
...
This
...
noncompliant
...
code
...
example
...
ignores
...
the
...
return
...
value
...
of
...
the
...
String.replace()
...
method,
...
failing
...
to
...
update
...
the
...
original
...
string.
...
The
...
String.replace()
...
method
...
cannot
...
modify
...
the
...
state
...
of
...
the
...
String
...
(because
...
String
...
objects
...
are
...
immutable);
...
rather,
...
it
...
returns
...
a
...
reference
...
to
...
a
...
new
...
String
...
object
...
containing the modified string.
Code Block | ||
---|---|---|
| ||
the desired result. {code:bgColor=#FFcccc} public class IgnoreReplace { public static void main(String[] args) { String original = "insecure"; original.replace( 'i', '9' ); System.out.println(original); } } {code} |
It
...
is
...
especially
...
important
...
to
...
process
...
the
...
return
...
values
...
of
...
immutable
...
object
...
methods.
...
Although many
...
methods
...
of
...
mutable
...
objects
...
operate
...
by
...
changing
...
some
...
internal
...
state
...
of
...
the
...
object,
...
methods
...
of
...
immutable
...
objects
...
cannot change
...
the
...
object
...
and
...
often
...
return
...
a
...
mutated new
...
object
...
,
...
leaving
...
the
...
original
...
object
...
unchanged.
...
Compliant
...
Solution
...
This
...
compliant
...
solution
...
correctly
...
updates
...
the
...
String
...
reference
...
original
...
with
...
the
...
return
...
value
...
from
...
the
...
String.replace()
method:
Code Block | ||
---|---|---|
| ||
}} method. {code:bgColor=#ccccff} public class DoNotIgnoreReplace { public static void main(String[] args) { String original = "insecure"; original = original.replace( 'i', '9' ); System.out.println(original); } } {code} h2. Risk Assessment Ignoring method return values can lead to unexpected program behavior. || Rule || Severity || Likelihood || Remediation Cost || Priority || Level || | EXP00-J | medium | probable | medium | {color:#cc9900}{*}P8{*}{color} | {color:#cc9900}{*}L2{*}{color} | h3. Automated Detection The Coverity Prevent Version 5.0 *CHECKED_RETURN* checker can detect the instance where Value returned from a function is not checked for errors before being used. h2. Related Guidelines | [seccode:CERT C Secure Coding Standard] | [seccode:EXP12-C. Do not ignore values returned by functions] | | [cplusplus:CERT C++ Secure Coding Standard] | [cplusplus:EXP12-CPP. Do not ignore values returned by functions or methods] | | [ISO/IEC TR 24772:2010|http://www.aitcnet.org/isai/] | "Passing Parameters and Return Values \[CSJ\]" | | [MITRE CWE|http://cwe.mitre.org/] | [CWE ID 252|http://cwe.mitre.org/data/definitions/252.html], "Unchecked Return Value" | h2. Bibliography |\[[API 2006|AA. Bibliography#API 06]\]| method [delete()|http://java.sun.com/javase/6/docs/api/java/io/File.html#delete()]| | | method [replace()|http://java.sun.com/javase/6/docs/api/java/lang/String.html#replace(char,%20char)]| |\[[Green 2008|AA. Bibliography#Green 08]\] |["String.replace"|http://mindprod.com/jgloss/gotchas.html]| |\[[Pugh 2009|AA. Bibliography#Pugh 09]\] |misusing putIfAbsent| ---- [!The CERT Oracle Secure Coding Standard for Java^button_arrow_left.png!|02. Expressions (EXP)] [!The CERT Oracle Secure Coding Standard for Java^button_arrow_up.png!|02. Expressions (EXP)] [!The CERT Oracle Secure Coding Standard for Java^button_arrow_right.png!|jg:EXP01-J. Do not confuse abstract object equality with reference equality] |
Risk Assessment
Ignoring method return values can lead to unexpected program behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP00-J | Medium | Probable | Medium | P8 | L2 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| JAVA.NULL.RET.UNCHECKED | Call Might Return Null (Java) | ||||||
Coverity | 7.5 | CHECKED_RETURN | Implemented | ||||||
Parasoft Jtest |
| CERT.EXP00.NASSIG CERT.EXP00.AECB | Ensure method and constructor return values are used Avoid "try", "catch" and "finally" blocks with empty bodies | ||||||
PVS-Studio |
| V6010, V6101 | |||||||
SonarQube |
| Return values from functions without side effects should not be ignored Return values should not be ignored when they contain the operation status code | |||||||
SpotBugs |
| RV_RETURN_VALUE_IGNORED | Implemented |
Related Guidelines
VOID EXP12-CPP. Do not ignore values returned by functions or methods | |
Passing Parameters and Return Values [CSJ] | |
CWE-252, Unchecked Return Value |
Bibliography
[API 2006] | |
Misusing | |
[Seacord 2015] |
...