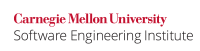
A nested class is any class whose declaration occurs within the body of another class or interface \[ [JLS 2005|AA. Bibliography#JLS 05]\]. The use of a nested class is error-prone unless the semantics are well understood. A common notion is that only the outer class can access the contents of the nested class. Not only does the nested class have access to the private fields of the outer class, the same fields can be accessed by another class within the package depending on whether the nested class is declared public or if it contains public methods or constructors. By default, the {{javac}} compiler converts the accessibility of private methods of a nested class to package-private. 2015]. The use of a nested class is error prone unless the semantics are well understood. A common notion is that only the nested class may access the contents of the outer class. Not only does the nested class have access to the private fields of the outer class, but the same fields can be accessed by any other class within the package when the nested class is declared public or if it contains public methods or constructors. As a result, the nested class must not expose the private members of the outer class to external classes or packages. Wiki Markup
According to The Java Language Specification (JLS), §8.3, "Field Declarations" [JLS 2015]: Also, according to the _Java Language Specification_ \[[JLS 2005|AA. Bibliography#JLS 05]\], [§8.3|http://java.sun.com/docs/books/jls/third_edition/html/classes.html#8.3] "Field Declarations" Wiki Markup
Note that a private field of a superclass might be accessible to a subclass (for example, if both classes are members of the same class). Nevertheless, a private field is never inherited by a subclass.
Noncompliant Code Example
This noncompliant code example exposes the sensitive private (x,y)
coordinates through the getPoint()
method of the inner class. Consequently, the AnotherClass
class that belongs to the same package can also access the coordinates.
Code Block | ||
---|---|---|
| ||
class Coordinates { private int x; private int y; public class Point { public void getPoint() { System.out.println("(" + x + "," + y + ")"); } } } class AnotherClass { public static void main(String[] args) { Coordinates c = new Coordinates(); Coordinates.Point p = c.new Point(); p.getPoint(); } } |
Compliant Solution
Use the private
access specifier for hiding to hide the inner class and all contained methods and constructors.
Code Block | ||
---|---|---|
| ||
class Coordinates { private int x; private int y; private class Point { private void getPoint() { System.out.println("(" + x + "," + y + ")"); } } } class AnotherClass { public static void main(String[] args) { Coordinates c = new Coordinates(); Coordinates.Point p = c.new Point(); // failsFails to compile p.getPoint(); } } |
Compilation of AnotherClass
now results in a compilation error because the class attempts to access a private nested class.
Risk Assessment
The Java language system weakens the accessibility of sensitive, private
entities in inner classesprivate members of an outer class when a nested inner class is present, which can result in a security weaknessan information leak.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
OBJ08-J |
Medium |
Probable |
Medium | P8 | L2 |
Automated Detection
Automated detection of non-private nested nonprivate inner classes that define non-private nonprivate members and constructors is straight-forward. However, this guideline applies only when those classes could potentially expose sensitive data or operations from the outer class. Detection of sensitive data or operations requires programmer assistance.that leak private data from the outer class is straightforward.
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| JAVA.CLASS.ICSBS | Inner Class Should be Static (Java) | ||||||
Parasoft Jtest |
| CERT.OBJ08.INNER | Make all member classes "private" |
Related Guidelines
...
...
, Use of Inner Class Containing Sensitive Data |
...
Bibliography
...
...
[ |
...
...
...
...
...
Section 2.3, "Inner Classes" | |
Securing Java: Getting Down to Business with Mobile Code |
...
Instances|http://java.sun.com/docs/books/jls/third_edition/html/classes.html#8.1.3] and [§8.3|http://java.sun.com/docs/books/jls/third_edition/html/classes.html#8.3] "Field Declarations" \[[Long 2005|AA. Bibliography#Long 05]\] §2.3, Inner Classes \[[McGraw 2000|AA. Bibliography#McGraw 00]\] Securing Java, Getting Down to Business with Mobile CodeOBJ12-J. Do not leak references to inner class objects when the outer class object maintains sensitive data 04. Object Orientation (OBJ) 05. Methods (MET)