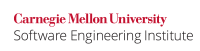
The C++ Standard, [filebuf], paragraph 2 [ISO/IEC 14882-2014], states the following:
The restrictions on reading and writing a sequence controlled by an object of class
basic_filebuf<charT, traits>
are the same as for reading and writing with the Standard C libraryFILE
s.
The C Standard, subclause Section 7.19.5.3 of C99 places the following restrictions on update streams: \[[, paragraph 6 [ISO/IEC 9899:1999|AA. Bibliography#ISO/IEC 9899-1999]\]
<blockquote><p>When a file is opened with update mode both input and output may be performed on the associated stream. However, output shall not be directly followed by input without an intervening call to the <code>fflush</code> function or to a file positioning function (<code>fseek</code>, <code>fsetpos</code>, or <code>rewind</code>), and input shall not be directly followed by output without an intervening call to a file positioning function, unless the input operation encounters end-of-file. Opening (or creating) a text file with update mode may instead open (or create) a binary stream in some implementations.</p></blockquote>Receiving input from a stream directly following an output to that stream without an intervening call to {{fflush()}}, {{fseek()}}, {{fsetpos()}}, or {{rewind()}}, or outputting to a stream after receiving input from it without a call to {{fseek()}}, {{fsetpos()}}, {{rewind()}} if the file is not at end-of-file results in [undefined behavior|BB. Definitions#undefined behavior]. Consequently, a call to {{fseek()}}, {{fflush()}} or {{fsetpos()}} is necessary between input and output to the same stream (see [FIO07-CPP. Prefer fseek() to rewind()|FIO07-CPP. Prefer fseek() to rewind()]). Wiki Markup
...
], places the following restrictions on FILE
objects opened for both reading and writing:
When a file is opened with update mode . . ., both input and output may be performed on the associated stream. However, output shall not be directly followed by input without an intervening call to the
fflush
function or to a file positioning function (fseek
,fsetpos
, orrewind
), and input shall not be directly followed by output without an intervening call to a file positioning function, unless the input operation encounters end-of-file.
Consequently, the following scenarios can result in undefined behavior:
- Receiving input from a stream directly following an output to that stream without an intervening call to
std::basic_filebuf<T>::seekoff()
if the file is not at end-of-file - Outputting to a stream after receiving input from that stream without a call to
std::basic_filebuf<T>::seekoff()
if the file is not at end-of-file
No other std::basic_filebuf<T>
function guarantees behavior as if a call were made to a standard C library file-positioning function, or std::fflush()
.
Calling std::basic_ostream<T>::seekp()
or std::basic_istream<T>::seekg()
eventually results in a call to std::basic_filebuf<T>::seekoff()
for file stream positioning. Given that std::basic_iostream<T>
inherits from both std::basic_ostream<T>
and std::basic_istream<T>
, and std::fstream
inherits from std::basic_iostream
, either function is acceptable to call to ensure the file buffer is in a valid state before the subsequent I/O operation.
Noncompliant Code Example
This noncompliant code example appends data to the end of a file and then reads from the same file.
Code Block | ||||
---|---|---|---|---|
| ||||
char data[BUFFERSIZE];
char append_data[BUFFERSIZE];
char *file_name;
FILE *file;
/* Initialize file_name */
file = fopen(file_name, "a+");
if (file == NULL) {
/* Handle error */
}
/* initialize append_data */
if (fwrite(append_data, BUFFERSIZE, 1, file) != BUFFERSIZE) {
/* Handle error */
}
if (fread(data, BUFFERSIZE, 1, file) != 0) {
/* Handle there not being data */
}
fclose(file);
|
However, because the stream is not flushed in between the call to fread()
and fwrite()
, the behavior is undefined.
Compliant Solution (FILE*
)
In this compliant solution, fseek()
is called in between the output and input, eliminating the undefined behaviorHowever, because there is no intervening positioning call between the formatted output and input calls, the behavior is undefined.
Code Block | ||||
---|---|---|---|---|
| ||||
char data[BUFFERSIZE]; char append_data[BUFFERSIZE]; char *file_name; FILE *file; /* initialize file_name */ file = fopen(file_name, "a+"); if (file == NULL#include <fstream> #include <string> void f(const std::string &fileName) { /* Handle error */ } /* Initialize append_data */ if (fwrite(append_data, BUFFERSIZE, 1, file) != BUFFERSIZE) { /* Handle error */ } if (fseek(file, 0L, SEEK_SET) != 0) { /* Handle error */ } if (fread(data, BUFFERSIZE, 1, file) != 0) { /* Handle there not being data */ } fclose(file); |
Noncompliant Code Example (iofstream
)
This noncompliant code example uses C++ iostreams, but makes the same mistake as the previous noncompliant code example.
Code Block | ||||
---|---|---|---|---|
| ||||
{ char data[BUFFERSIZE]; char append_data[BUFFERSIZE]; char *file_name; * Initialize file_name and append_data */ fstream file( file_name, fstream::in | fstream::out | fstream::app); file << append_data << endsstd::fstream file(fileName); if (!file.is_open()) { // Handle error return; } file << "Output some data"; std::string str; file >> data; // ... } /* File gets closed here */str; } |
Compliant Solution
...
In this compliant solution, the std::basic_istream<T>::seekg()
member function function is called in between the output and input, eliminating the undefined behavior.
Code Block | ||||
---|---|---|---|---|
| ||||
{ char data[BUFFERSIZE]; char append_data[BUFFERSIZE]#include <fstream> #include <string> void f(const std::string &fileName) { std::fstream file(fileName); charif *(!file_name; .is_open()) { * Initialize file_name and append_data */// Handle error fstream file( file_name, fstream::in | fstream::out | fstream::app); return; } file << "Output some append_data << ends; data"; std::string str; file.seekg(0, std::ios::beg); file >> data; // ... } /* File gets closed here */str; } |
Risk Assessment
Alternately inputting and outputting from a stream without an intervening flush or positioning call results in is undefined behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
FIO50-CPP |
Low |
Likely |
Medium | P6 | L2 |
Automated Detection
Fortify SCA Version 5.0 with CERT C Rule Pack can detect violations of this rule.
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Axivion Bauhaus Suite |
| CertC++-FIO50 | |||||||
CodeSonar |
| IO.IOWOP IO.OIWOP | Input After Output Without Positioning Output After Input Without Positioning | ||||||
Helix QAC |
| DF4711, DF4712, DF4713 | |||||||
| CERT_CPP-FIO50-a | Do not alternately input and output from a stream without an intervening flush or positioning call | |||||||
Polyspace Bug Finder |
| CERT C++: FIO50-CPP | Checks for alternating input and output from a stream without flush or positioning call (rule fully covered) |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
...
Related Guidelines
This rule appears in the C Secure Coding Standard as supplements FIO39-C. Do not alternately input and output from a stream without an intervening flush or positioning call.
Bibliography
...
[ |
...
...
...
1999] | Subclause 7.19.5.3, |
...
"The fopen Function" | |
[ISO/IEC 14882-2014] | Clause 27, "Input/Output Library" |
...
{{fopen}} function"FIO38-CPP. Do not use a copy of a FILE object for input and output 09. Input Output (FIO) FIO40-CPP. Reset strings on fgets() failure