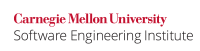
Assertions are a valuable diagnostic tool for finding and eliminating software defects that may result in vulnerabilities. (See see MSC11-C. Incorporate diagnostic tests using assertions). ) The runtime assert()
macro has some limitations, however, in that it incurs a runtime overhead and because it calls abort()
. Consequently, the runtime assert()
macro is useful only for identifying incorrect assumptions and not for runtime error checking. As a result, runtime assertions are generally unsuitable for server programs or embedded systems.
...
Code Block |
---|
static_assert(constant-expression, string-literal); |
Section Subclause 6.7.10 of the C Standard [ISO/IEC 9899:2011] states:
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <assert.h> struct timer { unsigned char MODE; unsigned int DATA; unsigned int COUNT; }; int func(void) { assert(sizeof(struct timer) == sizeof(unsigned char) + sizeof(unsigned int) + sizeof(unsigned int)); } |
Although the use of the runtime assertion is better than nothing, it needs to be placed in a function and executed. This means that it is usually far away from the definition of the actual structure to which it refers. The diagnostic occurs only at runtime and only if the code path containing the assertion is executed.
...
For assertions involving only constant expressions, a preprocessor conditional statement may be used, as in this examplecompliant solution:
Code Block | ||||
---|---|---|---|---|
| ||||
struct timer { unsigned char MODE; unsigned int DATA; unsigned int COUNT; }; #if (sizeof(struct timer) != (sizeof(unsigned char) + sizeof(unsigned int) + sizeof(unsigned int))) #error "Structure must not have any padding" #endif |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <assert.h>
struct timer {
unsigned char MODE;
unsigned int DATA;
unsigned int COUNT;
};
static_assert(sizeof(struct timer) == sizeof(unsigned char) + sizeof(unsigned int) + sizeof(unsigned int),
"Structure must not have any padding");
|
...
Other uses of static assertion are shown in STR07-C. Use the bounds-checking interfaces for remediation of existing string manipulation code and FIO35FIO34-C. Use feof() and ferror() to detect end-of-file and file errors when sizeof(int) == sizeof(char)Distinguish between characters read from a file and EOF or WEOF.
Risk Assessment
Static assertion is a valuable diagnostic tool for finding and eliminating software defects that may result in vulnerabilities at compile time. The absence of static assertions, however, does not mean that code is incorrect.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
DCL03-C |
Low |
Unlikely |
High | P1 | L3 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Axivion Bauhaus Suite |
| CertC-DCL03 | |||||||
Clang |
| misc-static-assert | Checked by clang-tidy | ||||||
CodeSonar |
| (customization) | Users can implement a custom check that reports uses of the assert() macro | ||||||
Compass/ROSE |
Could detect violations of this rule merely by looking for calls to | |||||||
ECLAIR |
|
CC2.DCL03 | Fully implemented | ||||||||
LDRA tool suite |
| 44 S | Fully |
implemented |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
C++ Secure Coding Standard | VOID DCL03-CPP. Use a static assertion to test the value of a constant expression |
Bibliography
[Becker 2008] |
[Eckel 2007] |
[ISO/IEC 9899:2011] |
Subclause 6.7.10, "Static Assertions" |
[Jones 2010] |
[Klarer 2004] |
[Saks 2005] |
[Saks 2008] |
...
...