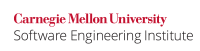
This standard recommends the inclusion of diagnostic tests into your program using the assert()
macro or other mechanisms (see MSC11-A. Incorporate diagnostic tests using assertions). Static assertion is a new facility in the C++ 0X draft standard. This facility gives the ability to make assertions at compile time rather than runtime, providing the following advantages:
- all processing must be performed during compile time – no runtime cost in space or time is tolerable
- assertion failure must result in a meaningful and informative diagnostic error message
- it can be used at file or block scope
- misuse does not result in silent malfunction, but rather is diagnosed at compile time
Static assertions take the form:
static_assert(constant-expression, string-literal);
In a static assert declaration the constant-expression
is a constant expression that can be contextually converted to bool
. If the value of the expression when converted is true, the declaration has no effect. Otherwise the program is ill-formed, and a diagnostic message (which includes the text of the string-literal
is issued at compile time. For example:
static_assert(sizeof(int) <= sizeof(long), "sizeof(int) <= sizeof(long)"); /* Passes */ static_assert(sizeof(double) <= sizeof(int), "sizeof(double) <= sizeof(int)"); /* Fails */
Non-Compliant Code Example
This non-compliant code uses the assert()
macro to assert a property concerning a memory-mapped structure that is essential for the code that uses this structure to behave correctly.
struct timer { uint8_t MODE; uint32_t DATA; uint32_t COUNT; }; int main(void) { assert(offsetof(timer, DATA) == 4); }
While the use of the runtime assertion is better than nothing, it needs to be placed in a function and executed, typically removed from the actual structure to which it refers. The diagnostic only occurs at runtime, and only if the code path containing the assertion is executed.
Compliant Solution
For assertions involving only constant expressions, some implementations allow the use a preprocessor conditional statement, as in:
struct timer { uint8_t MODE; uint32_t DATA; uint32_t COUNT; }; #if (offsetof(timer, DATA) != 4) #error DATA must be at offset 4 #endif
Using #error
directives allows for clear diagnostic messages. Because this approach evaluates assertions at compile time, there is no run-time penalty.
Unfortunately, this solution is not portable. C99 does not require that implementations support sizeof
, offsetof
or enumeration constants in #if
conditions. According to Section 6.10.1, "Conditional inclusion", all identifiers in the expression that controls conditional inclusion either are, or are not, macro names. Some compilers allow these constructs in conditionals as an extension, but most do not.
Compliant Solution
This compliant solution mimics the behavior of static_assert
in a portable manner.
#define JOIN(x, y) JOIN_AGAIN(x, y) #define JOIN_AGAIN(x, y) x ## y #define static_assert(e, s) \ typedef char JOIN(assertion_failed_at_line_, __LINE__) [(e) ? 1 : -1] struct timer { uint8_t MODE; uint32_t DATA; uint32_t COUNT; }; static_assert(offsetof(struct timer, DATA) == 4, "offsetof(struct timer, DATA) == 4");
The static_assert()
macro accepts a constant expression e
which is evaluated as the first operand to the conditional operator. If e
evaluates to nonzero, an array type with a dimension of 1 is defined; otherwise an array type with a dimension of -1 is defined. Because it is invalid to declare an array with a negative dimension, the resulting type definition will be flagged by the compiler. The name of the array is used to indicate the location of the failed assertion.
The macro argument string-literal
is ignored in this case, this is meant for future compatibility.
The JOIN()
macro used the ##
operator [[ISO/IEC 9899-1999]] to concatenate tokens. See [[PRE05-A. Understand macro replacement when concatenating tokens]] to understand how macro replacement behaves in C when using the ##
operator.
Risk Assessment
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL03-A |
1 (low) |
1 (unlikely) |
1 (high) |
P1 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 6.10.1, "Conditional inclusion," and Section 6.10.3.3, "The ## operator"
[Klarer 04] R. Klarer, J. Maddock, B. Dawes, and H. Hinnant. "Proposal to Add Static Assertions to the Core Language (Revision 3)" (ISO C++ committee paper ISO/IEC JTC1/SC22/WG21/N1720, October 2004). This document is available online at http://www.open-std.org/jtc1/sc22/wg21/docs/papers/2004/n1720.html.
[Saks 08] Dan Saks, Stephen C. Dewhurst. Presentation. Sooner Rather Than Later: Static Programming Techniques for C++.
[Saks 05] Dan Saks. _Catching errors early with compile-time assertions. Embedded Systems Design. June, 2005.
[Eckel 2007] Bruce Eckel. Thinking in C++ - Volume 2. January 25, 2007.
DCL02-A. Use visually distinct identifiers 02. Declarations and Initialization (DCL) DCL04-A. Take care when declaring more than one variable per declaration