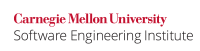
If an environment variable might have changed during program execution, get a fresh copy using getenv()
. If you rely on your old variable, you could be left with incorrect data or a dangling pointer.
Non-Compliant Coding Example
After a call to setenv()
, environment pointers to the old value and copies of the old value will be incorrect.
char *temp; char *copy; if ((temp = getenv("TEST_ENV")) != NULL) { copy = malloc(strlen(temp) + 1); if (copy != NULL) { strcpy(copy, temp); } else { /* handle error condition */ } } /* ...program code... */ setenv("TEST_ENV", var, 1); /* ...program code... */ printf("TEST_ENV: %s\n", temp); printf("TEST_ENV: %s\n", copy);
Neither of the print statements will be correct.
Compliant Solution
You should always fetch fresh copies of environment variables, especially if you know that a value has changed.
char *temp;
char *copy;
if ((temp = getenv("TEST_ENV")) != NULL)
else {
/* handle error condition */
}
}
/* ...program code... */
setenv("TEST_ENV", var, 1);
/* ...program code... */
if ((temp = getenv("TEST_ENV")) != NULL) {
copy = malloc(strlen(temp) + 1);
if (copy != NULL)
}
else
}
printf("TEST_ENV: %s\n", temp);
printf("TEST_ENV: %s\n", copy);
This will provide us with the current value of the environment variable.
Risk Assessment
The program would not be using current environment values, causing unexpected results.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ENV31-C |
1 (low) |
1 (low) |
3 (low) |
P3 |
L3 |
References
[[ISO/IEC 9899-1999:TC2]] Section 7.20.4, "Communication with the environment"