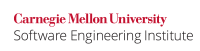
Under many hosted environments it is possible to access the environment through a modified form of main
:
main(int argc, char **argv, char **envp)
According to C99 [[ISO/IEC 9899-1999:TC2]]:
In a hosted environment, the main function receives a third argument, char *envp[], that points to a null-terminated array of pointers to char, each of which points to a string that provides information about the environment for this execution of the program.
However, using the setenv()
or putenv()
functions might cause environment memory to be reallocated, leaving envp
pointing to the wrong location. To illustrate:
extern char **environ; int main(int argc, char **argv, char **envp) { printf("environ: %p\n", environ); printf("envp: %p\n", envp); putenv("MY_NEW_VAR=new_value", 1); printf("--Added MY_NEW_VAR--\n"); printf("environ: %p\n", environ); printf("envp: %p\n", envp); }
Yields:
% ./envp-environ environ: 0xbf8656ec envp: 0xbf8656ec --Added MY_NEW_VAR-- environ: 0x804a008 envp: 0xbf8656ec
If you need to directly access or manipulate the environment, it is safer to use environ
.
Non-Compliant Coding Example
After a call to setenv()
, envp
may not have the proper environment values.
int main(int argc, char **argv, char **envp) { setenv("MY_NEW_VAR", "new_value", 1); if (envp != NULL) { for (i = 0; envp[i] != NULL; i++) { printf("%s\n", envp[i]); } } return 0; }
MY_NEW_VAR
will not be found in envp
.
Compliant Solution (POSIX)
Use environ
in place of envp
.
extern char **environ; int main(int argc, char **argv) { setenv("MY_NEW_VAR", "new_value", 1); if (environ != NULL) { for (i = 0; environ[i] != NULL; i++) { printf("%s\n", environ[i]); } } return 0; }
Note: if you have a great deal of unsafe envp
code, you could save time in your remediation by aliasing. Change:
main(int argc, char **argv, char **envp)
To:
extern char **environ; #define envp environ main(int argc, char **argv)
Risk Assessment
The program would not be using current environment values, causing unexpected results.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ENV31-C |
1 (low) |
1 (low) |
3 (low) |
P3 |
L3 |
References
[[ISO/IEC 9899-1999:TC2]] Section J.5.1, "Environment Arguments"