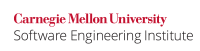
Declaring function arguments const
indicates that the function promises not to change these values.
In C, function arguments are passed by value rather than by reference. Thus, while a function may change the values passed in, these changed values are forgotten once the function exits. For this reason, most programmers assume a function will not change its arguments, and declaring them const
is unnecessary.
void foo(int x) { x = 3; /* only lasts until end of function */ /* ... */ }
Pointers themselves are similar. A function may change a pointer to point to a different object, or NULL, yet that change will be forgotten once the function exits. Thus, declaring a pointer as const
is unnecessary.
void foo(int* x) { x = NULL; /* only lasts until end of function */ /* ... */ }
But pointed-to values are another matter. A function may modify a value referenced by a pointer argument, with the modification being retained after the function exits.
void foo(int* x) { if (x != NULL) { *x = 3; /* visible outside function */ } /* ... */ }
If a function does not modify the pointed-to value, it should declare this value as const
. This improves code readability and consistency.
Risk Assessment
Not declaring an unchanging value const
prohibits the function from working with values already cast as const
. One could sidestep this problem by typecasting away the const
, but that violates EXP05-A. Do not cast away a const qualification.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP10-A |
1 (medium) |
1 (unlikely) |
2 (high) |
P2 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
EXP09-A. Ensure pointer arithmetic is used correctly 03. Expressions (EXP) EXP30-C. Do not depend on order of evaluation between sequence points