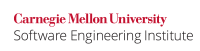
Information determined prior to program startup from the hosted environment is supplied to the program. This information includes command line arguments and environmental variables.
Command line arguments are passed as arguments to main()
. In the following definition for main()
the array members argv[0]
through argv[argc-1]
inclusive contain pointers to strings.
int main(int argc, char *argv[]) { /* ... */ }
If the value of argc
is greater than zero, the string pointed to by argv[0]
represents the program name. If the value of argc is greater than one, the strings pointed to by argv[1]
through argv[argc-1]
represent the program parameters.
The getenv()
function searches an environment list, provided by the host environment, for a string that matches the string pointed to by name. The set of environment names and the method for altering the environment list are implementation-defined.
Non-compliant Code Example 1
The contents of argv[0]
can be manipulated by an attacker to cause a buffer overflow in the following program:
int main(int argc, char *argv[]) { ... char prog_name[128]; strcpy(prog_name, argv[0]); ... }
Non-compliant Code Example 2
Reading environment variables into fixed length arrays can also result in a buffer overflow.
char buff[256]; strcpy(buff, (char *)getenv("EDITOR"));
Compliant Solution 1
The strlen()
function should be used to determine the length of the strings referenced by argv[0]
through argv[argc-1]
so that adequate memory can be dynamically allocated:
int main(int argc, char *argv[]) { ... char * prog_name = (char *)malloc(strlen(argv[0])+1); if (prog_name != NULL) { strcpy(prog_name, argv[1]); } else { /* Couldn't get the memory - recover */ } ... }
Compliant Solution 2
The strlen()
function should be used to determine the length of environmental variables so that adequate memory can be dynamically allocated:
char *editor; char *buff; editor = (char *)getenv("EDITOR"); if (editor) { buff = (char *)malloc(strlen(editor)+1); strcpy(buff, editor); }
References
- ISO/IEC 9899-1999 5.1.2.2.1 Program startup
- ISO/IEC 9899-1999 7.20.4.5 The getenv function