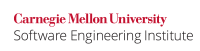
The behavior of a program is undefined when it uses the value of a pointer to a FILE
object after the associated file is closed (see undefined behavior 148.) Programs that close the standard streams (especially stdout
but also stderr
and stdin
) must be careful not to use the stream objects in subsequent function calls, particularly those that implicitly operate on such objects (such as printf()
, perror()
, and getc()
).
This rule can be generalized to other representations of files, such as an int
representing a POSIX file descriptor that has been passed to close()
..
Noncompliant Code Example
In this noncompliant code example the printf()
function is called after the stdout
stream is closed.
#include <stdio.h> int close_stdout(void) { if (fclose(stdout) == EOF) { return -1; } printf("stdout successfully closed.\n"); return 0; }
Compliant Solution
In this compliant solution, stdout
is not used again after it is closed. This must remain true for the remainder of the program.
#include <stdio.h> int close_stdout(void) { if (fclose(stdout) == EOF) { return -1; } fprintf(stderr, "stdout successfully closed.\n"); return 0; }
Risk Assessment
Using the value of a pointer to a FILE
object after the associated file is closed is undefined behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
FIO46-C | Medium | Unlikely | Medium | P4 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
5.0 | Can detect violations of this rule with CERT C Rule Pack | ||
2024.4 | RH.LEAK | ||
9.7.1 | 49 D | Fully implemented |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Bibliography
[IEEE Std 1003.1:2013] | XSH, System Interfaces, open |
[ISO/IEC 9899:2011] | Subclause 7.21.3, "Files" Subclause 7.21.5.1, "The |