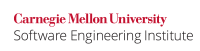
Using type definitions (typedef
) can often improve code readability. However, type definitions to pointer types can make it more difficult to write const-correct code because the const qualifier will be applied to the pointer type, not the underlying declared type.
Noncompliant Code Example
The following type definition improves readability at the expense of introducing a const-correctness issue.
struct obj { int i; float f; }; typedef struct obj *ObjectPtr; void func(const ObjectPtr o);
Compliant Solution
This compliant solution makes use of type definitions, but does not declare a pointer type and so cannot be used in a const-incorrect manner.
struct obj { int i; float f; }; typedef struct obj Object; void func(const Object *o);
Using type definitions (typedef
) can often improve code readability. However, type definitions to pointer types can make it more difficult to write const-correct code because the const qualifier will be applied to the pointer type, not the underlying declared type.
Noncompliant Code Example (Windows)
The Win32 SDK headers make use of type definitions for most of the types involved in Win32 APIs, but the following noncompliant solution demonstrates a const-correctness bug.
#include <Windows.h> void func(const LPSTR str) { /* Use, but do not mutate, str */ }
Compliant Solution (Windows)
The compliant solution demonstrates a common naming convention found in the Win32 APIs to utilize the proper type.
#include <Windows.h> void func(LPCSTR str) { /* Use, but do not mutate, str */ }
Noncompliant Code Example (Windows)
Note that many structures in the Win32 API are declared with pointer type definitions, but not pointer to const type definitions (LPPOINT, LPSIZE, et al). In these cases, it is suggested to create your own type definition from the base structure type.
#include <Windows.h> void func(const LPPOINT pt) { }
Compliant Code Example (Windows)
#include <Windows.h> typedef const POINT *LPCPOINT; void func(LPCPOINT pt) { }
Exceptions
Function pointer types are an exception to this recommendation.
Noncompliant Code Example
The following declaration of the signal()
function is difficult to read and comprehend.
void (*signal(int, void (*)(int)))(int);
Compliant Solution
This compliant solution makes use of type definitions to specify the same type as in the noncompliant code example.
typedef void (*SighandlerType)(int signum); extern SighandlerType signal( int signum, SighandlerType handler );
Risk Assessment
Code readability is important for discovering and eliminating vulnerabilities.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
DCL05-C | low | unlikely | medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Compass/ROSE |
|
|
|
9.7.1 | 299 S | Fully implemented | |
PRQA QA-C | Unable to render {include} The included page could not be found. | Secondary Analysis | Fully implemented |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.