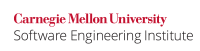
Declaring function arguments const
indicates that the function promises not to change these values.
In C, function arguments are passed by value rather than by reference. While a function may change the values passed in, these changed values are discarded once the function returns. For this reason, most programmers assume a function will not change its arguments, and declaring them const
is unnecessary.
void foo(int x) { x = 3; /* only lasts until end of function */ /* ... */ }
Pointers behave in a similar fashion. A function may change a pointer to reference a different object, or NULL, yet that change is discarded once the function exits. Consequently, declaring a pointer as const
is unnecessary.
void foo(int* x) { x = NULL; /* only lasts until end of function */ /* ... */ }
Non-Compliant Code Example
Unlike passed-by-value arguments and pointers, pointed-to values are a concern. A function may modify a value referenced by a pointer argument, with the modification being retained after the function exits.
void foo(int* x) { if (x != NULL) { *x = 3; /* visible outside function */ } /* ... */ }
Non-Compliant Code Example
If a function modifies a pointed-to value, declaring this value as const
will be caught by the compiler.
void foo(const int * x) { if (x != NULL) { *x = 3; /* generates compiler warning */ } /* ... */ }
Compliant Solution
If a function does not modify the pointed-to value, it should declare this value as const
. This improves code readability and consistency.
void foo(const int * x) { if (x != NULL) { printf("Value is %d\n", *x); } /* ... */ }
Non-Compliant Code Example
This non-compliant code example, defines a fictional version of the standard strcat()
function called strcat_nc()
. This function differs from strcat()
in that the second argument is not const
-qualified.
char *strcat_nc(char *s1, char *s2); char *str1 = "str1"; const char *str2 = "str2"; char str3[] = "str3"; const char str4[] = "str4"; strcat_nc(str3, str2); strcat_nc(str1, str3); strcat_nc(str4, str3);
The function would behave the same as strcat()
, but the compiler generates warnings in incorrect locations, and fails to generate them in correct locations.
In the first strcat_nc()
call, the compiler will generate a warning about attempting to cast away const on str2
. This is a good warning, as strcat_nc()
does not modify its second argument, yet fails to declare it const
.
In the second strcat_nc()
call, the compiler will happily compile the code with no warnings, but the resulting code will attempt to modify the "str1"
literal, which may be impossible; the literal may not be defined in the heap. This violates STR05-A. Use pointers to const when referencing string literals.
In the final strcat_nc()
call, the compiler generates a warning about ateempting to cast away const on str4
. This is a valid warning.
Compliant Solution
This compliant solution uses the prototype for the strcat()
from C90. Although the restrict
type qualifier did not exist in C90, const
did. In general, the arguments should be declared in a manner consistent with the semantics of the function. In the case of strcat()
, the initial argument can be changed by the function while the second argument cannot.
char *strcat(char *s1, const char *s2); char *str1 = "str1"; const char *str2 = "str2"; char str3[] = "str3"; const char str4[] = "str4"; strcat(str3, str2); strcat(str1, str3); /* Note: reversed args */ strcat(str4, str3); /* different 'const' qualifiers */
The const
-qualification of the second argument s2
eliminates the spurious warning in the initial invocation, but maintains the valid warning on the final invocation in which a const
-qualified object is passed as the first argument (which can change). Finally, the middle strcat()
invocation is now valid, as str1
is a valid destination string, as the string exists on the stack and may be safely modified.
Risk Assessment
Not declaring an unchanging value const
prohibits the function from working with values already cast as const
. One could sidestep this problem by typecasting away the const
, but that violates EXP05-A. Do not cast away a const qualification.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL13-A |
medium |
unlikely |
high |
P2 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]]
[[ISO/IEC PDTR 24772]] "CSJ Passing parameters and return values"
DCL12-A. Create and use abstract data types 02. Declarations and Initialization (DCL)