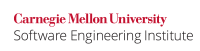
Bit-fields can be used to allow flags or other integer values with small ranges to be packed together to save storage space.
It is implementation-defined whether the specifier int
designates the same type as signed int
or the same type as unsigned int
for bit-fields. C99 integer promotions also requires that "If an int
can represent all values of the original type, the value is converted to an int
; otherwise, it is converted to an unsigned int
."
In the following example:
struct { unsigned int a: 8; } bits = {255}; int main(void) { printf("unsigned 8-bit field promotes to %s.\n", (bits.a - 256 > 0) ? "signed" : "unsigned"); }
The type of the expression (bits.a - 256 > 0)
is compiler dependent and may be either signed or unsigned depending on the compiler implementor's interpretation of the standard.
The first interpretation is that when bits.a
is used as an rvalue, the type is "unsigned int
" as declared. An unsigned int
cannot be represented as an int
, so integer promotions require that this be an unsigned int
, and consequently "unsigned".
The second interpretation is that bits.a
is an 8-bit integer. As a result, this eight bit value can be represented as an int
, so integer promotions require that it be converted to int
, and consequently "signed".
The type of the bit-field when used in an expression also has implications for long
and long long
types. Compilers that follow the second interpretation of the standard and determine the size from the width of the bit-field will promote values of these types to int
. For example, gcc interprets the following as an eight bit value and promote it to int
:
struct { unsigned long long a:8; } ull = {255};
Risk Assessment
Making invalid assumptions about the type of a bit-field or its layout can result in unexpected program flow.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT12-A |
1 (low) |
1 (unlikely) |
2 (medium) |
P2 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 6.7.2, "Type specifiers"