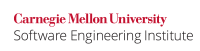
The C Standard function rand
(available in stdlib.h
) does not have good random number properties. The numbers generated by rand
have a comparatively short cycle, and the numbers may be predictable. To achieve the best random numbers possible, an implementation-specific function needs to be used.
Non-Compliant Code Example
The following code generates an ID with a numeric part produced by calling the rand()
function. The IDs produced will be predictable and have limited randomness.
enum {len = 12}; char id[len]; /* id will hold the ID, starting with the characters "ID" */ /* followed by a random integer */ int r; int num; /* ... */ r = rand(); /* generate a random integer */ num = snprintf(id, len, "ID%-d", r); /* generate the ID */ /* ... */
Compliant Solution (BSD)
A better pseudo random number generator is the BSD function random()
.
enum {len = 12}; char id[len]; /* id will hold the ID, starting with the characters "ID" */ /* followed by a random integer */ int r; int num; /* ... */ srandom(time(0)); /* seed the PRNG with the current time */ /* ... */ r = random(); /* generate a random integer */ num = snprintf(id, len, "ID%-d", r); /* generate the ID */ /* ... */
The rand48
family of functions provides another alternative.
Note. These pseudo random number generators use mathematical algorithms to produce a sequence of numbers with good statistical properties, but the numbers produced are not genuinely random. For additional randomness, Linux users can use the character devices /dev/random
or /dev/urandom
, but it is advisable to retrieve only a small number of characters from these devices. (The device /dev/random
may block for a long time if there are not enough events going on to generate sufficient randomness; /dev/urandom
does not block.)
Risk Assessment
Using the rand
function may lead to programming problems (for example, non-unique unique IDs) or weak cryptography.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC30-C |
1 (low) |
1 (unlikely) |
1 (high) |
P1 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 7.20.2.1, "The rand function"