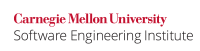
This standard recommends the inclusion of diagnostic tests into your program using the assert()
macro or other mechanisms (see [[MSC11-A. Incorporate diagnostic tests using assertions]]). Static assertion is a new facility in the C++ )X draft standard. This facility gives the ability to make assertions at compile time rather than runtime, providing the following advantages:
- all processing must be performed during compile time – no runtime cost in space or time is tolerable
- assertion failure must result in a meaningful and informative diagnostic error message
- it can be used at file or block scope
- misuse does not result in silent malfunction, but rather is diagnosed at compile time
Static assertions take the form"
static_assert(constant-expression, string-literal);
In a static assert declaration the constant-expression
is a constant expression that can be contextually converted to bool. If the value of the expression when converted is true, the declaration has no effect. Otherwise the program is ill-formed, and a diagnostic message (which includes the text of the string-literal
is issued at compile time.
While not yet available in C, this behavior can be mimicked as follows:
#define static_assert(constant-expression, string-literal) \ do { typedef int a[(constant-expression) ? 1 : -1]; } while(0) int main(void) { static_assert(sizeof(int) <= sizeof(long)); /* Passes */ static_assert(sizeof(double) <= sizeof(int)); /* Fails */ }
The macro argument string-literal
is ignored in this case, this is meant for future compatibility.
Non-Compliant Code Example
This non-compliant code example ...
Compliant Solution
This compliant solution ...
static_assert(sizeof(long) >= 8, "64-bit code generation required for this library.");
Risk Assessment
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL03-A |
1 (low) |
1 (unlikely) |
1 (high) |
P1 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section ???, "Name"
[Klarer 04] R. Klarer, J. Maddock, B. Dawes, and H. Hinnant. "Proposal to Add Static Assertions to the Core Language (Revision 3)" (ISO C++ committee paper ISO/IEC JTC1/SC22/WG21/N1720, October 2004). This document is available online at http://www.open-std.org/jtc1/sc22/wg21/docs/papers/2004/n1720.html.
DCL02-A. Use visually distinct identifiers 02. Declarations and Initialization (DCL) DCL04-A. Take care when declaring more than one variable per declaration