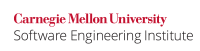
C++2004, section 15.3 "Handling an Exception", says:
The handlers for a try block are tried in order of appearance. That makes it possible to write handlers that can never be executed, for example by placing a handler for a derived class after a handler for a corresponding base class.
A ... in a handlers exception-declaration functions similarly to ... in a function parameter declaration; it specifies a match for any exception. If present, a ... handler shall be the last handler for its try block.
Consequently if two handlers catch exceptions that are derived from the same base class (such as std::exception
), the most derived exception should come first.
Non-Compliant Code Example
In this non-compliant code example, the first handler will catch all exceptions of class B
, as well as exceptions of class D
, since they are also of class B
. Consequently the second handler will not catch any exceptions.
// classes used for exception handling class B {}; class D: public B {}; // ... Using the classes from above try { // ... } catch (B &b) { // ... } catch (D &d) { // ... }
Compliant Solution
In this compliant solution, the first handler will catch all exceptions of class D
, and the second handler will catch all the other exceptions of class B
.
// classes used for exception handling class B {}; class D: public B {}; // ... Using the classes from above try { // ... } catch (D &d) { // ... } catch (B &b) { // ... }
Risk Assessment
Badly ordering exception handlers can cause unexpected control flow.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ERR36-CPP |
high |
likely |
low |
P9 |
L1 |
Bibliography
[ISO/IEC 14882-2003]
[MISRA 08] Rule 15-3-6 & 15-3-7
ERR35-CPP. A handler in a constructor or destructor's function-try-block should not reference class data 12. Exceptions and Error Handling (ERR) ERR37-CPP. A function with an exception-specification should not throw any other exceptions