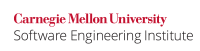
Programs are forbidden to lock on an object of a class that implements either or both of the Lock
and Condition
interfaces of the java.util.concurrent.locks
package. Using the intrinsic locks of these classes is a questionable practice even in cases where the code may appear to function correctly. This problem generally arises when code is refactored from intrinsic locking to the java.util.concurrent
dynamic-locking utilities.
Noncompliant Code Example (ReentrantLock
Lock Object)
The doSomething()
method in this noncompliant code example synchronizes on the intrinsic lock of an instance of ReentrantLock
rather than on the reentrant mutual exclusion Lock
encapsulated by ReentrantLock
.
private final Lock lock = new ReentrantLock(); public void doSomething() { synchronized(lock) { // ... } }
Compliant Solution (lock()
and unlock()
)
This compliant solution uses the lock()
and unlock()
methods provided by the Lock
interface.
private final Lock lock = new ReentrantLock(); public void doSomething() { lock.lock(); try { // ... } finally { lock.unlock(); } }
In the absence of a requirement for the advanced functionality of the java.util.concurrent
package's dynamic-locking utilities, it is better to use the Executor
framework or other concurrency primitives such as synchronization and atomic classes.
Risk Assessment
Synchronizing on the intrinsic lock of high-level concurrency utilities can cause nondeterministic behavior because the class can end up with two different locking policies.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
LCK03-J |
medium |
probable |
medium |
P8 |
L2 |
Related Vulnerabilities
Any vulnerabilities resulting from the violation of this rule are listed on the CERT website.
Bibliography
TODO check references
[[API 2006]] |
|
[[Findbugs 2008]] |
|
[[Pugh 2008]] |
"Synchronization" |
[[Miller 2009]] |
Locking |
[[Tutorials 2008]] |
null [!The CERT Oracle Secure Coding Standard for Java^button_arrow_up.png!] null