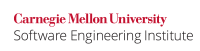
Synchronizing a class is unnecessary when it is designed for single-threaded use. Such classes are required to document their lack of thread-safety. For instance, the documentation of class java.lang.StringBuilder
states [[API 06]] :
This class is designed for use as a drop-in replacement for
StringBuffer
in places where the string buffer was being used by a single thread (as is generally the case). Where possible, it is recommended that this class be used in preference toStringBuffer
as it will be faster under most implementations.
Multithreaded clients of classes that are not thread-safe must externally synchronize any accesses if the documentation of the classes specify the lack of thread-safety, or fail to provide any conclusive information.
Classes that use mutable static
fields must always internally synchronize accesses to their fields. This is because there is no guarantee that all clients will synchronize externally when accessing the field. Because a static
field is shared by all clients, unrelated clients may violate the contract by not performing adequate synchronization.
Noncompliant Code Example
This noncompliant code example does not synchronize access to the static
field counter
.
final class CountHits { private static int counter; public void incrementCounter() { counter++; } }
It relies on clients to externally synchronize the object and specifies its unsafe behavior in the documentation. However, there is no guarantee that all unrelated (trusted or untrusted) clients will follow this advice.
Compliant Solution
This compliant solution internally synchronizes the counter
field and consequently, does not depend on any external synchronization.
final class CountHits { private static int counter; public synchronized void incrementCounter() { counter++; } }
Risk Assessment
Failing to internally synchronize classes containing accessible static members can result in unexpected results when a client fails to obey the classes' synchronization policy.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON32- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]]
[[Bloch 08]] Item 67: "Avoid excessive synchronization"
VOID CON06-J. Do not defer a thread that is holding a lock 11. Concurrency (CON)