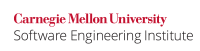
According to the Java Language Specification [[JLS 05]], section, 8.3.1.4 volatile
Fields:
A field may be declared volatile, in which case the Java memory model (§17) ensures that all threads see a consistent value for the variable.
Notably, this applies only to fields and not to the contents of arrays that are declare volatile
. A thread may not observe a recent write to the array's element from another thread.
Noncompliant Code Example
This noncompliant code example shows an array that is declared volatile
. It appears that, when a value is written by a thread to one of the array elements, it will be visible to other threads immediately. This is counter-intuitive as the volatile
keyword just makes the array reference visible to all threads and not the actual data contained within the array.
class Unsafe { private volatile int[] arr = new int[20]; // other code } arr[2] = 10;
Compliant Solution
This compliant solution suggests using the java.util.concurrent.atomic.AtomicIntegerArray
concurrency utility. Using its set(index, value)
method ensures that the write is atomic and the resulting value is immediately visible to other threads. The other threads can retrieve a value from a specific index by using the get(index)
method.
class Safe { AtomicIntegerArray air = new AtomicIntegerArray(5); // other code } air.set(1, 10);
Risk Assessment
Assuming that the contents of a an array declared volatile
, are volatile
can lead to stale reads.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON03-J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]]
CON02-J. Facilitate thread reuse by using Thread Pools 08. Concurrency (CON) CON04-J. Do not subclass Thread if you can use a Runnable instead