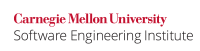
A switch
block comprises several case
labels and an optional but highly recommended default
label. By convention, statements that follow each case label end with a break
statement, responsible for transferring the control to the end of the switch
block. When omitted, the statements in the subsequent case
label are executed. Because the break
statement is optional, its omission produces no compiler warnings. If this behavior is unintentional, it can cause unexpected control flow.
Noncompliant Code Example
In this noncompliant code example, the case wherein the card
is 11, does not have a break
statement. As a result, the statements for card = 12
are also executed.
int card = 11; switch (card) { /* ... */ case 11: System.out.println("Jack"); case 12: System.out.println("Queen"); break; case 13: System.out.println("King"); break; default: System.out.println("Invalid Card"); break; }
Compliant Solution
This compliant solution terminates each case (including the default
case) with a break
statement.
int card = 11; switch (card) { /* ... */ case 11: System.out.println("Jack"); break; case 12: System.out.println("Queen"); break; case 13: System.out.println("King"); break; default: System.out.println("Invalid Card"); break; }
Exceptions
EX1: The last label in a switch
statement does not require a break
statement. The break
statement serves to skip to the end of the switch
block, so control transfers to statements following the switch
block irrespective of its presence. Conventionally, the last label is the default
label.
EX2: When it is required to execute the same code for multiple cases, it is permissible to omit the break
statement. However, these instances must be explicitly documented.
int card = 11; int value; // Cases 11,12,13 fall through to the same case switch (card) { // MSC13-J:EX2: these three cases are treated identically case 11: case 12: case 13: value = 10; break; default: // Handle Error Condition }
EX3: A case needs no break
statement if its last statement is a return
or throw
.
Risk Assessment
Failure to include break
statements may cause unexpected control flow.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC14- J |
medium |
unlikely |
low |
P6 |
L2 |
Other Languages
This rule appears in the C Secure Coding Standard as MSC17-C. Finish every set of statements associated with a case label with a break statement.
This rule appears in the C++ Secure Coding Standard as MSC18-CPP. Finish every set of statements associated with a case label with a break statement.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 2005]] Section 14.11 The switch Statement
MSC13-J. Do not modify the underlying collection when an iteration is in progress 49. Miscellaneous (MSC) MSC15-J. Use numerical comparison operators to terminate a loop whose counter changes by more than one