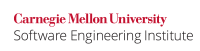
A constructor in the base class can reference uninitialized fields or potentially cause other damage while calling an overridable method. This is because, it is possible for the wrong version (in a sub class) of the method to get invoked.
Noncompliant Code Example
This noncompliant example invokes the doLogic
method from the constructor. The super class doLogic
method gets invoked on the first call, however, the overriding method is invoked on the second one. In the second call, the issue is that the constructor for SubClass
initiates the super class's constructor which undesirably ends up calling SubClass
's doLogic()
method. The value of color
is left as null
because the initialization of the BaseClass
has not yet concluded.
class BaseClass { public BaseClass() { doLogic(); } public void doLogic() { System.out.println("This is super-class!"); } } class SubClass extends BaseClass { private String color = null; public SubClass() { super(); color = "Red"; } public void doLogic() { System.out.println("This is sub-class! The color is :" + color); //color becomes null //other operations } } public class Overridable { public static void main(String[] args) { BaseClass bc = new BaseClass(); //prints "This is super-class!" BaseClass sc = new SubClass(); //prints "This is sub-class! The color is :null" } }
Compliant Solution
This compliant solution declares the doLogic
method as final
so that it is no longer overridable.
class BaseClass { public BaseClass() { doLogic(); } public final void doLogic() { System.out.println("This is super-class!"); } }
Risk Assessment
Allowing a constructor to call overridable methods may give an attacker access to this
before an object is fully initialized which, in turn, could lead to a vulnerability.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MET32-J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Chapter 8, Classes
[[SCG 07]] Guideline 4-3 Prevent constructors from calling methods that can be overridden
MET31-J. Ensure that hashCode() is overridden when equals() is overridden 09. Methods (MET) 10. Exceptional Behavior (EXC)