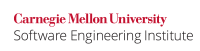
Java input classes, for example Scanner
and BufferedInputStream
, often buffer the underlying input stream to facilitate fast, non-blocking I/O.
As the InputStream
class is abstract
, a wrapper such as BufferedInputStream
is required to provide a concrete implementation that overrides its methods. It is permissible to create multiple wrappers on an InputStream
. Programs that encourage multiple wrappers around the same stream, however, behave significantly different depending on whether the InputStream
allows look-ahead or not. An adversary can exploit this difference in behavior by, for example, redirecting System.in
(from a file). This is also possible when a program uses the System.setIn()
method to redirect System.in
. That said, redirecting input from the console is a standard practice in UNIX based platforms but finds limited application in others such as Windows, where console programs are largely considered outmoded. In general, any input stream that supports non-blocking buffered I/O is susceptible to misuse.
Do not create multiple wrappers that buffer input from an InputStream
. Instead, create and use only one wrapper, either by passing it as an argument to the methods that need it or declaring it as a class variable.
Noncompliant Code Example
Despite just one declaration, this noncompliant code example creates multiple BufferedInputStream
wrappers on System.in
because each time getChar()
is called, it conceives a new BufferedInputStream
. Because of the inherent channeling and buffering mechanism, the data that is read from the underlying stream once, cannot be replaced so that a second call can read the same data again. While this code uses a BufferedInputStream
to illustrate that any buffered wrapper is unsafe, this condition is also exploitable if a Scanner
is used instead.
public final class InputLibrary { public static char getChar() throws EOFException { BufferedInputStream in = new BufferedInputStream(System.in); // wrapper int input = in.read(); if (input == -1) { throw new EOFException(); } // down casting is permitted because InputStream guarantees read() in range // 0..255 if it is not -1 return (char)input; } public static void main(String[] args) { try { // Either redirect input from the console or use // System.setIn(new FileInputStream("input.dat")); System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.out.println("ERROR"); } } }
Implementation Details
This program was compiled with the command javac InputLibrary.java
on a system with Java 1.6.0. When run from the command line with java InputLibrary
, the program successfully takes two characters as input and prints them out. However, when run with java InputLibrary < input
, where input
is a file that contains the exact same input, the program prints "ERROR" because the second call to getChar()
finds no characters to read upon encountering the end of the stream.
Compliant Solution
Create and use only a single BufferedInputStream
on System.in
. This compliant solution declares the BufferedInputStream
as a class variable so that all methods can access it. However, if a program were to use this library in conjunction with other input from a user that needs another buffered wrapper on System.in
, the library must be modified so that all code uses the same buffered wrapper instead of additional ones that are created.
public final class InputLibrary { private static BufferedInputStream in = new BufferedInputStream(System.in); public static char getChar() throws EOFException { int input = in.read(); if (input == -1) { throw new EOFException(); } in.skip(1); // This statement is now necessary to go to the next line // The Noncompliant code example deceptively worked without it return (char)input; } public static void main(String[] args) { try { System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.out.println("ERROR"); } } }
It may appear that the mark()
and reset()
methods of BufferedInputStream
would replace the read bytes but this idea is deceptive, for, these methods provide look-ahead by operating on the internal buffers and not directly on the underlying stream.
Implementation Details
This program was compiled with the command javac InputLibrary.java
on a system with Java 1.6.0. When run from the command line with java InputLibrary
, the program successfully takes two characters as input and prints them out. Also, when run with java InputLibrary < input
, where input
is a file that contains the exact same input, the program successfully takes two characters as input and print them out.
Risk Assessment
Creating multiple buffered wrappers around an InputStream
can cause unexpected program behavior when the InputStream
is re-directed.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO36- J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] method read
[[API 06]] class BufferedInputStream
FIO35-J. Always validate user input 09. Input Output (FIO) 09. Concurrency (CON)