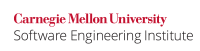
In the absence of autoboxing, the values of boxed primitives cannot be compared using the ==
and !=
operators by default. This is because these are interpreted as reference comparison operators. This condition is demonstrated in the first noncompliant code example.
Autoboxing on the other hand, can also produce subtle effects. It works by automatically wrapping the primitive type to the corresponding wrapper object. Some care should be taken during this process, especially when performing comparisons. The Java Language Specification [[JLS 05]] explains this point clearly:
If the value
p
being boxed istrue
,false
, abyte
, achar
in the range\u0000
to\u007f
, or anint
orshort
number between-128
and127
, then letr1
andr2
be the results of any two boxing conversions ofp
. It is always the case thatr1 == r2
.
Noncompliant Code Example
This noncompliant example (adopted from [[Bloch 09]]), defines a Comparator
with a compare()
method. The compare()
method accepts two boxed primitives as arguments. Note that primitive integers are also accepted by this declaration as they are appropriately autoboxed. The main issue is that the ==
operator is being used to compare the two boxed primitives. This however, compares their references and not the actual values.
static Comparator<Integer> cmp = new Comparator<Integer>() { public int compare(Integer i, Integer j) { return i < j ? -1 : (i == j ? 0 : 1); } };
Compliant Solution
To be compliant, use any of the four comparison operators <, >, <= and >=. The ==
and !=
operators should not be used to compare boxed primitives.
public int compare(Integer i, Integer j) { return i < j ? -1 : (i > j ? 1 : 0) ; }
Noncompliant Code Example
This code uses ==
to compare two Integer
objects. According to EXP03-J. Do not compare String objects using equality or relational operators, for ==
to return true
for two object references, they must point to the same underlying object. Results of using the ==
operator in this case will be misleading.
public class TestWrapper2 { public static void main(String[] args) { Integer i1 = 100; Integer i2 = 100; Integer i3 = 1000; Integer i4 = 1000; System.out.println(i1==i2); System.out.println(i1!=i2); System.out.println(i3==i4); System.out.println(i3!=i4); } }
These comparisons generate the output sequence: true
, false
, false
and true
. The cache
in the Integer
class can only make the integers from -127
to 128
refer to the same object, which explains the output of the above code. To avoid making such mistakes, use equals
instead of ==
to compare wrapper classes (See EXP03-J for further details).
Compliant Solution
Using object1.equals(object2) only compares their values. Now, the results will be true
, as expected.
public class TestWrapper2 { public static void main(String[] args) { Integer i1 = 100; Integer i2 = 100; Integer i3 = 1000; Integer i4 = 1000; System.out.println(i1.equals(i2)); System.out.println(i3.equals(i4)); } }
Noncompliant Code Example
Sometimes a list of integers is desired. Recall that the type parameter inside the angle brackets of a list cannot be of a primitive type. It is not possible to form an ArrayList<int>
. With the help of the wrapper classs and autoboxing, storing primitive integer values in ArrayList<Integer>
becomes possible.
In this noncompliant code example, it is desired to count the integers of arrays list1
and list2
. As class Integer
only caches integers from -127 to 128, when an int
value is beyond this range, it is autoboxed into the corresponding wrapper type. The ==
operator returns false
when these distinct wrapper objects are compared. As a result, the output of this example is 0.
import java.util.ArrayList; public class Wrapper { public static void main(String[] args) { // Create an array list of integers, where each element // is greater than 127 ArrayList<Integer> list1 = new ArrayList<Integer>(); for(int i=0;i<10;i++) list1.add(i+1000); // Create another array list of integers, where each element // is the same as the first list ArrayList<Integer> list2 = new ArrayList<Integer>(); for(int i=0;i<10;i++) list2.add(i+1000); int counter = 0; for(int i=0;i<10;i++) if(list1.get(i) == list2.get(i)) counter++; // print the counter System.out.println(counter); } }
If it were possible to expand the Integer
cache (for example, caching all the values -32K – 32K, which means that all the int
values in the example would be autoboxed to cached Integer
objects), then the results may have differed.
Compliant Solution
This compliant solution uses the equals()
method for performing comparisons of wrapped objects. It produces the correct output 10.
public class TestWrapper1 { public static void main(String[] args) { // Create an array list of integers, where each element // is greater than 127 ArrayList<Integer> list1 = new ArrayList<Integer>(); for(int i=0;i<10;i++) list1.add(i+1000); // Create another array list of integers, where each element // is the same as the first one ArrayList<Integer> list2 = new ArrayList<Integer>(); for(int i=0;i<10;i++) list2.add(i+1000); int counter = 0; for(int i=0;i<10;i++) if(list1.get(i).equals(list2.get(i))) counter++; System.out.println(counter); } }
Risk Assessment
Using the equal and not equal operators to compare boxed primitives can lead to erroneous comparisons.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP32- J |
low |
likely |
medium |
P6 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Bloch 09]] 4. "Searching for the One"
[[Pugh 09]] Using == to compare objects rather than .equals
EXP31-J. Avoid side effects in assertions 04. Expressions (EXP) 05. Scope (SCP)