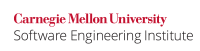
Most methods perform no security manager checks because they do not provide access to sensitive parts of the system, such as the filesystem. Most methods that do provide security manager checks check that every class and method in the call stack is authorized before they proceed. This allows restricted programs, such as Java applets, to have full access to the core Java library. This security model prevents a sensitive method from acting on behalf of a malicious method that hides behind a trusted method in the call stack.
However, certain methods use a reduced security check, that only checks that the calling method has access, rather then checking every method in the call stack. Code that invokes these methods must guarentee that they are not being invoked on behalf of untrusted code. These methods are:
Methods that check calling method only |
---|
|
|
|
|
|
|
|
|
Because the java.lang.reflect.Field.setAccessible/getAccessible
methods are used to instruct the JVM to override the language access checks, they perform standard (and more restrictive) security manager checks and consequently lack the vulnerability discussed in this guideline. Nevertheless, these methods should be used only with extreme caution. The remaining set*
and get*
field reflection methods perform only the language access checks and consequently are vulnerable.
Class loaders allow a Java application to be dynamically extended at runtime by loading classes. For each class that is loaded, the JVM tracks the class loader that was used to load the class. When a loaded class first refers to another class, the virtual machine requests that the referenced class be loaded by the same class loader used to load the referencing class. Java's class loader architecture controls interaction between code loaded from different sources by allowing the use of different class loaders. This separation of class loaders is fundamental to the separation of code; it prevents malicious code from gaining access to and subverting trusted code.
Several methods, that are charged with loading classes, delegate their work to the class loader of the class of the method that called them. The security checks associated with loading classes is often performed by class loaders. Consequently, any method that invokes one of these class-loading methods must guarentee that these methods are not acting on behalf of untrusted code. These methods are:
Methods that use calling method's class loader |
---|
|
|
|
|
|
|
|
|
|
|
|
|
In practice, the trusted code's class loader frequently allows these methods to be invoked whereas untrusted code's class loader may lack these privileges. However, when the untrusted code's class loader delegates to the trusted code's class loader, the untrusted code gains visibility to the trusted code. In the absence of such a delegation relationship, the class loaders would ensure namespace separation; consequently, the untrusted code would be unable to observe members or to invoke methods belonging to the trusted code.
A problem arises because the class loader delegation model is fundamental to many Java implementations and frameworks. So the best advice is to avoid exposing the methods listed above to untrusted code. Consider, for example, an attack scenario where untrusted code is attempting to load a privileged class. If its class loader is permitted to delegate the class loading to a trusted class's class loader, privilege escalation can occur, because the untrusted code's class loader may lack permission to load the requested privileged class on its own. Furthermore, if the trusted code accepts tainted inputs, the trusted code's class loader could load additional privileged — or even malicious — classes on behalf of the untrusted code.
Classes that have the same defining class loader will exist in the same namespace but can have different privileges, depending on the security policy. Security vulnerabilities can arise when trusted code coexists with untrusted code (or less-privileged code) that was loaded by the same class loader. In this case, the untrusted (or less-privileged) code can freely access members of the trusted code according to their declared accessibility. When the trusted code uses any of the tabulated APIs, it bypasses security manager checks (with the exception of loadLibrary()
and load()
).
With the exception of the loadLibrary()
and load()
methods, the tabulated methods fail to perform any security manager checks. The loadLibrary()
and load()
APIs are typically used from within a doPrivileged
block; in that case, unprivileged callers can directly invoke them without requiring any special permissions. Consequently, the security manager checks are curtailed at the immediate caller. The security manager fails to examine the entire call stack and so fails to enhance security. Accepting tainted inputs from untrusted code and allowing them to be used by these APIs may expose vulnerabilities.
This guideline is an instance of SEC03-J. Do not load trusted classes after allowing untrusted code to load arbitrary classes. Many examples also violate SEC00-J. Do not allow privileged blocks to leak sensitive information across a trust boundary.
Noncompliant Code Example
In this noncompliant code example a call to System.loadLibrary()
is embedded in a doPrivileged
block. This is insecure because a library can be loaded on behalf of untrusted code. In essence, the untrusted code's class loader may be able to indirectly load a library even though it lacks sufficient permissions to do so directly. After loading the library, untrusted code can call native methods from the library if those methods are accessible. This is possible because the doPrivileged
block stops security manager checks being applied to callers further up the execution chain.
public void load(String libName) { AccessController.doPrivileged(new PrivilegedAction() { public Object run() { System.loadLibrary(libName); return null; } }); }
Nonnative library code can also be susceptible to related security flaws. Loading a nonnative safe library may not directly expose a vulnerability. However, after loading an additional unsafe library, an attacker can easily exploit the safe library if it contains other vulnerabilities. Moreover, nonnative libraries often use doPrivileged
blocks, making them attractive targets.
Compliant Solution
This compliant solution hard-codes the name of the library to prevent the possibility of tainted values. It also reduces the accessibility of method load()
from public
to private
. Consequently, untrusted callers are prohibited from loading the awt
library.
private void load() { AccessController.doPrivileged(new PrivilegedAction() { public Object run() { System.loadLibrary("awt"); return null; } }); }
Noncompliant Code Example
A method that passes untrusted inputs to the Class.forName()
method might permit an attacker to access classes with escalated privileges. The single argument Class.forName()
method is another API method that uses its immediate caller's class loader to load a requested class. Untrusted code can misuse this API to indirectly manufacture classes that have the same privileges as those of the attacker's immediate caller.
public Class loadClass(String className) { // className may be the name of a privileged or even a malicious class return Class.forName(className); }
Compliant Solution
This compliant solution hard-codes the class's name.
public Class loadClass() { return Class.forName("Foo"); }
Noncompliant Code Example
This noncompliant code example returns an instance of java.sql.Connection
from trusted to untrusted code. Untrusted code that lacks the permissions required to create a SQL connection can bypass these restrictions by using the acquired instance directly.
public Connection getConnection(String url, String username, String password) { // ... return DriverManager.getConnection(url, username, password); }
Compliant Solution
The getConnection()
method is unsafe because it uses the url
to indicate a class to be loaded; this class serves as the database driver. This compliant solution prevents a malicious user from supplying their own URL to the database connection; thereby limiting their ability to load untrusted drivers.
private String url = // hardwired value public Connection getConnection(String username, String password) { // ... return DriverManager.getConnection(this.url, username, password); }
Noncompliant Code Example (CVE-2013-0422)
Java 1.7.0u10 was exploited in January 2013 because of several vulnerabilities. One vulnerability in the MBeanInstantiator
class granted unprivileged code the ability to access any class regardless of the current security policy or accessibility rules. The MBeanInstantiator.findClass()
method could be invoked with any string and would attempt to return a Class
object. This method delegated its work to the loadClass()
method, whose source code is shown:
/** * Load a class with the specified loader, or with this object * class loader if the specified loader is null. **/ static Class<?> loadClass(String className, ClassLoader loader) throws ReflectionException { Class<?> theClass; if (className == null) { throw new RuntimeOperationsException(new IllegalArgumentException("The class name cannot be null"), "Exception occurred during object instantiation"); } try { if (loader == null) loader = MBeanInstantiator.class.getClassLoader(); if (loader != null) { theClass = Class.forName(className, false, loader); } else { theClass = Class.forName(className); } } catch (ClassNotFoundException e) { throw new ReflectionException(e, "The MBean class could not be loaded"); } return theClass; }
This method delegates the task of dynamically loading the specified class to the Class.forName()
method. The forName()
method delegates the work of loading the class to its calling method's class loader. Since the calling method was MBeanInstantiator.loadClass()
, the core class loader is used, which provides no security checks.
Compliant Solution (CVE-2013-0422)
Oracle mitigated this vulnerability in Java 1.7.0p11 by adding an access check to the loadClass()
method. This access check ensures that the caller is permitted to access the class being sought:
// ... if (className == null) { throw new RuntimeOperationsException(new IllegalArgumentException("The class name cannot be null"), "Exception occurred during object instantiation"); } ReflectUtil.checkPackageAccess(className); try { if (loader == null) // ...
Noncompliant Code Example (CERT Vul# 636312)
CERT Vulnerability 636312 describes a vulnerability in Java that was successfully exploited in August 2012. (The exploit actually used two vulnerabilities; the other one is described in SEC05-J. Do not use reflection to increase accessibility of classes, methods, or fields.
The exploit runs as an applet. The applet class loader ensures that an applet cannot directly invoke methods of classes present in the com.sun.*
package. A security manager check ensures that specific actions are allowed or denied depending on the privileges of all of the caller methods on the call stack (the privileges are associated with the code source that encompasses the class).
One goal of the exploit code was to access the private sun.awt.SunToolkit
class. However, invoking class.forName()
directly would cause a SecurityException
to be thrown. Consequently, the exploit code contains the following method to get any class, bypassing the security manager:
private Class GetClass(String paramString) throws Throwable { Object arrayOfObject[] = new Object[1]; arrayOfObject[0] = paramString; Expression localExpression = new Expression(Class.class, "forName", arrayOfObject); localExpression.execute(); return (Class)localExpression.getValue(); }
The {[java.beans.Expression.execute()}} method delegates its work to the following method:
private Object invokeInternal() throws Exception { Object target = getTarget(); String methodName = getMethodName(); if (target == null || methodName == null) { throw new NullPointerException((target == null ? "target" : "methodName") + " should not be null"); } Object[] arguments = getArguments(); if (arguments == null) { arguments = emptyArray; } // Class.forName() won't load classes outside // of core from a class inside core. Special // case this method. if (target == Class.class && methodName.equals("forName")) { return ClassFinder.resolveClass((String)arguments[0], this.loader); } // ...
The com.sun.beans.finder.ClassFinder.resolveClass()
method delegates its work to the findClass()
method:
public static Class<?> findClass(String name) throws ClassNotFoundException { try { ClassLoader loader = Thread.currentThread().getContextClassLoader(); if (loader == null) { loader = ClassLoader.getSystemClassLoader(); } if (loader != null) { return Class.forName(name, false, loader); } } catch (ClassNotFoundException exception) { // use current class loader instead } catch (SecurityException exception) { // use current class loader instead } return Class.forName(name); }
While this method is called in the context of an applet, it uses Class.forName()
to obtain the requested class. And Class.forName()
delegates the search to the calling method's class loader. In this case the calling class (com.sun.beans.finder.ClassFinder
) is part of core Java, so the trusting class loader is used, instead of the more paranoid applet class loader.
Compliant Solution (CVE-2012-4681)
Oracle mitigated this vulnerability by patching the com.sun.beans.finder.ClassFinder.findClass()
method. The checkPackageAccess()
method checks the entire call stack to ensure that Class.forName()
in this instance only fetches classes for trusted methods.
public static Class<?> findClass(String name) throws ClassNotFoundException { checkPackageAccess(name); try { ClassLoader loader = Thread.currentThread().getContextClassLoader(); if (loader == null) { // can be null in IE (see 6204697) loader = ClassLoader.getSystemClassLoader(); } if (loader != null) { return Class.forName(name, false, loader); } } catch (ClassNotFoundException exception) { // use current class loader instead } catch (SecurityException exception) { // use current class loader instead } return Class.forName(name); }
Applicability
Allowing untrusted code to invoke methods with reduced security checks can grant excessive abilities to malicious code. Likewise, allowing untrusted code to carry out actions using the immediate caller's class loader may allow the untrusted code to execute with the same privileges as the immediate caller.
This guideline does not apply to methods that do not use the immediate caller's class loader instance. For example, the three-argument java.lang.Class.forName()
method requires an explicit argument that specifies the class loader instance to use. Do not use the immediate caller's class loader as the third argument when instances must be returned to untrusted code.
public static Class forName(String name, boolean initialize, ClassLoader loader) /* explicitly specify the class loader to use */ throws ClassNotFoundException
Related Guidelines
[SCG 2010] | |
[SCG 2010] | Guideline 9-10: Be aware of standard APIs that perform Java language access checks against the immediate caller |
Bibliography
Oracle Security Alert for CVE-2013-0422
Manion, Art, Anatomy of Java Exploits, CERT/CC Blog, January 15, 2013 2:00 PM
Guillardoy, Esteban (Immunity Products), Java 0-day analysis (CVE-2012-4681), August 28, 2012
[Chan 1999] java.lang.reflect AccessibleObject