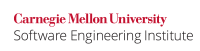
Nested classes are a broad set of classes that are classified as static member and inner classes. "An inner class is a nested class that is not explicitly or implicitly declared static." [JLS 05 Section 8.1.3, Inner Classes and Enclosing Instances]. An inner class may be local, anonymous or non-static.
Nested class usage is prone to error unless the semantics are well understood. A common notion is that only the outer class can access the contents of the nested inner class(es). Not only does the nested class have access to the private fields of the outer class, the same fields can be accessed by another class in the package depending on whether the nested class is declared public or if it contains public methods/constructors.
Noncompliant Code Example
The code in this noncompliant example illegally exposes the (x,y)
coordinates through the getPoint()
method of the inner class. The AnotherClass
class can as a result illegally access the coordinates which is clearly not desired.
class Coordinates { private int x; private int y; public class Point { public void getPoint() { System.out.println("(" + x + "," + y + ")"); } } } class AnotherClass { public static void main(String[] args) { Coordinates c = new Coordinates(); Coordinates.Point p = c.new Point(); p.getPoint(); } }
Compliant Solution
Use the private
access specifier for declaring the inner class(es) and all contained methods and constructors. The compiler will refuse to compile AnotherClass
because of its attempt to access a private nested class.
class Coordinates { private int x; private int y; private class Point { private void getPoint() { System.out.println("(" + x + "," + y + ")"); } } } class AnotherClass { public static void main(String[] args) { Coordinates c = new Coordinates(); Coordinates.Point p = c.new Point(); // fails to compile p.getPoint(); } }
Risk Assessment
The Java Language System weakens the access of private entities in inner classes which may result in a security weakness.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SCP02-J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Section 8.1.3, Inner Classes and Enclosing Instances
[[McGraw 00]]
[[Long 05]] Section 2.3, Inner Classes
SCP01-J. When developing Java clients (applets, mobile) assume that all scope can be overridden or modified 03. Scope (SCP) SCP03-J. Do not reuse names