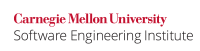
Cookies are an essential part of any web application; they are used for many purposes, including user authentication. A cookie is a small piece of data that is set by a web server's response that will be stored for a certain period of time on the client's computer. After a cookie has been set, all of the information within is sent in all subsequent requests to the cookie domain. Consequently, the information within a cookie is insecure; it is vulnerable to cross-site scripting (XSS) or man-in-the-middle attacks (among others). Servers must ensure that cookies lack excess or sensitive information about users. A partial list of such information includes user names, passwords, password hashes, credit cards, and any personally identifiable information about the user.
Noncompliant Code Example
In this noncompliant code example, the servlet stores the user name in the cookie to identify the user for authentication purposes.
import java.util.ArrayList; import java.util.List; import javax.servlet.http.*; import com.insecure.model.UserDAO; import com.insecure.databeans.UserBean; public class InsecureServlet extends HttpServlet { private UserDAO userDAO; // ... private String login(HttpServletRequest request, HttpServletResponse response) { List<String> errors = new ArrayList<String>(); request.setAttribute("errors", errors); Â Â Â Â Â Â Â String username = request.getParameter("username"); char[] password = request.getParameter("password").toCharArray(); Â Â Â Â Â Â // Basic input validation if(!username.matches("[\\w]*") || !password.toString().matches("[\\w]*")) { errors.add("Incorrect user name or password format."); return "error.jsp"; } Â Â Â Â Â UserBean dbUser = this.userDAO.lookup(username); if(!dbUser.checkPassword(password)) { errors.add("Passwords do not match."); return "error.jsp"; } Â Â Â // Create a cookie that contains the username Cookie userCookie = new Cookie("username", username); // Create a cookie that contains the password Cookie passCookie = new Cookie("password", password); // Add the cookie information to the response that the client will receive response.addCookie(userCookie); response.addCookie(passCookie); // Clear password char array Arrays.fill(password, ' '); return "welcome.jsp"; } }
Note that the noncompliant code example stores the user name and password within two cookie objects, which are sent to the client to be stored in a cookie. This code example is insecure because an attacker can discover this information by performing a cross-site scripting attack or by sniffing packets. Once the attacker gains access to the username and password, he can freely log in to the user's account. Even if the application had stored only the user name within the cookie for authentication purposes, an attacker could still use the user name to forge his own cookie and consequently bypass the authentication system.
Compliant Solution
This compliant solution stores user information using the HttpSesssion
class within the javax.servlet.http
package. Because HttpSession
objects are server-side, an attacker cannot use cross-site scripting or man-in-the-middle attacks to directly gain access to the session information. Rather, the cookie stores a session id that refers to the user's HttpSession
object stored on the server. Consequently, the attacker cannot gain access to the user's account details without first gaining access to the session id.
public class InsecureServlet extends HttpServlet { private UserDAO userDAO; // ... private String login(HttpServletRequest request) { List<String> errors = new ArrayList<String>(); request.setAttribute("errors", errors); String username = request.getParameter("username"); char[] password = request.getParameter("password").toCharArray(); // Basic input validation if(!username.matches("[\\w]*") || !password.toString().matches("[\\w]*")) { errors.add("Incorrect user name or password format."); return "error.jsp"; } UserBean dbUser = this.userDAO.lookup(username); if(!dbUser.checkPassword(password)) { errors.add("Passwords do not match."); return "error.jsp"; } HttpSession session = request.getSession(); // Invalidate old session id session.invalidate(); // Generate new session id session = request.getSession(true); // Set session timeout to one hour session.setMaxInactiveInterval(60*60); // Store user bean within the session session.setAttribute("user", dbUser.getUsername()); // Clear password char array Arrays.fill(password, ' '); return "welcome.jsp"; } }
This solution also invalidates the current session and creates a new session to avoid session fixation attacks; see [SD:OWASP 2009]. The solution also reduces the window in which an attacker could perform a session hijacking attack by setting the session timeout to one.
Risk Assessment
Violation of this guideline places sensitive information within cookies, making the information vulnerable to packet sniffing or cross-site scripting attacks.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO15-J |
medium |
probable |
medium |
P8 |
L2 |
Bibliography
[SD:OWASP 2009] Session Fixation in Java
[SD:OWASP 2010] Cross-site Scripting
[SD:Oracle 2010] javax.servlet.http Package API
The World Wide Web Security FAQ