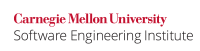
Methods return values to communicate failure or success and at other times, to update the caller's objects or fields. Security risks can arise if return values are simply ignored or if suitable action is not taken on their receipt. Return values may be ignored intentionally or even unintentionally. For example, when getter methods that return a value are named after an action, such as ProcessBuilder.redirectErrorStream()
, a programmer may not realize that a return value is expected. Incidentally, the only purpose of the redirectErrorStream()
method is to tell using a return value, whether the process builder merges standard error and standard output. The action of actually redirecting the error stream is performed by its overloaded single argument version. It is important to read the API documentation so that return values are not ignored.
Noncompliant Code Example
This noncompliant code example attempts to delete a file, but does not check whether the operation has succeeded.
File someFile = new File("someFileName.txt"); // do something with someFile someFile.delete();
Compliant Solution
This compliant solution checks the (boolean
) value returned by the delete()
method and, if necessary, handles the error.
File someFile = new File("someFileName.txt"); // do something with someFile if (!someFile.delete()) { // handle the fact that the file has not been deleted }
Noncompliant Code Example
This noncompliant code example ignores the return value of the String.replace
method. As a result, the original string is not updated even though it seems otherwise. The String.replace()
method does not modify the state of the String
but instead, returns a reference to a new String
object with the replacements in place.
public class Ignore { public static void main(String[] args) { String original = "insecure"; original.replace( 'i', '9' ); System.out.println(original); } }
Compliant Solution
This compliant solution correctly updates the original
String
object by assigning to it the return value.
public class DoNotIgnore { public static void main(String[] args) { String original = "insecure"; original = original.replace( 'i', '9' ); System.out.println(original); } }
Another source of security vulnerabilities caused by ignoring return values is detailed in FIO02-J. Keep track of bytes read and account for character encoding while reading data.
Risk Assessment
Ignoring method return values can lead to unanticipated program behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP02- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Secure Coding Standard as EXP12-C. Do not ignore values returned by functions.
This rule appears in the C++ Secure Coding Standard as EXP12-CPP. Do not ignore values returned by functions or methods.
References
[[API 06]] method delete()
[[API 06]] method replace()
[[Green 08]] "String.replace"
[[Pugh 09]] misusing putIfAbsent
[[MITRE 09]] CWE ID 252 "Unchecked Return Value"
EXP01-J. Ensure a null pointer is not dereferenced 04. Expressions (EXP) EXP03-J. Do not compare String objects using equality or relational operators