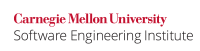
Methods return values to signify failure or success or, at other times, to update the caller's objects or fields. Security risks can arise if return values are simply ignored or if suitable action is not taken on their receipt.
Noncompliant Code Example
This noncompliant code example attempts to delete a file, but does not check that the operation succeeds.
File someFile = new File("someFileName.txt"); // do something with someFile someFile.delete();
Compliant Solution
In the compliant solution, the (boolean
) value returned by the delete()
method is checked and, if necessary, the error is handled.
File someFile = new File("someFileName.txt"); // do something with someFile if (!someFile.delete()) { // handle the fact that the file has not been deleted }
Noncompliant Code Example
This noncompliant code example ignores the return value while making use of the String.replace
method. As a result, the original string is not updated even though it seems otherwise.
public class Ignore { public static void main(String[] args) { String original = "insecure"; original.replace( 'i', '9' ); System.out.println(original); } }
Compliant Solution
The compliant solution correctly updates the original
string object by assigning to it the return value.
public class DoNotIgnore { public static void main(String[] args) { String original = "insecure"; original = original.replace( 'i', '9' ); System.out.println(original); } }
Another source of coding bugs caused by ignoring return values is detailed in FIO03-J. Keep track of bytes read and account for character encoding while reading data.
Risk Assessment
Ignoring method return values may lead to erroneous computation which, in turn, may lead to vulnerabilities.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP02-J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Secure Coding Standard as EXP12-C. Do not ignore values returned by functions.
This rule appears in the C++ Secure Coding Standard as EXP12-CPP. Do not ignore values returned by functions or methods.
References
[[API 06]] method delete()
[[API 06]] method replace()
[[Green 08]] "String.replace"
[[Pugh 09]] misusing putIfAbsent
[[MITRE 09]] CWE ID 252 "Unchecked Return Value"
EXP00-J. Use the same type for the second and third operands in conditional expressions 02. Expressions (EXP) EXP03-J. Do not compare String objects using equality or relational operators