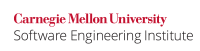
The enhanced for
statement introduced in Java 1.5, commonly referred to as the for-each idiom, is primarily used for iterating over collections of objects. While similar to the for
statement, assignments to the loop variable do not modify the collection of objects over which the loop iterates. Assignments to the loop variable may not have the effect intended by the developer and should be avoided.
As detailed in the Java Language Specification [[JLS 05]] section 14.14.2, "The enhanced for
statement", an enhanced for
statement of the form:
for (ObjType obj : someIterableItem) { // ... }
is equivalent to a standard for
loop of the form:
for (Iterator myIterator = someIterableItem.iterator(); iterator.hasNext();) { ObjType obj = myIterator.next(); // ... }
Consequently, an assignment to the loop variable is equivalent to modifying a variable local to the loop body, whose initial value is the object that the loop iterator refers to. While this modification is not necessarily erroneous, it may obscure the loop functionality or indicate a misunderstanding of the underlying implementation of the enhanced for
statement.
It is recommended that all enhanced for
statement loop variables be declared final
. The final
declaration causes Java compilers to flag and reject any assignments made to the loop variable, in the loop body.
Noncompliant Code Example
This noncompliant code example attempts to initialize a Character
array using an enhanced for
loop. However, because assignments to the loop variable do not modify the array over which the loop iterates, the array is not suitably initialized.
Character[] array = new Character[10]; for(Character c: array) c = 'x'; // initialization attempt for(int i=0;i<array.length;i++) System.out.print(array[i]); // prints 10 "null"s
Note that if c
is declared final
, a compiler error results when an attempt is made to initialize it.
Compliant Solution
This compliant solution correctly initializes the array using a for
loop.
Character[] array = new Character[10]; for(int i=0;i<array.length;i++) array[i] = 'x'; for(final Character c: array) System.out.print(c); // prints 10 "x"s
Risk Assessment
Attempts to assign to the loop variable from within the enhanced for
loop (for-each idiom) are futile and may leave the class in a fragile, inconsistent state.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL30- J |
low |
unlikely |
low |
P3 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
TODO
References
[[JLS 05]] Section 14.14.2 "The enhanced for statement"
DCL08-J. Enforce compile-time type checking of variable argument types 03. Declarations and Initialization (DCL) DCL31-J. Qualify mathematical constants with the static and final modifiers