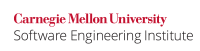
A switch
block comprises several case labels and an optional but highly recommended default
label. By convention, statements that follow each case label end with a break
statement, responsible for transferring the control to the end of the switch block. When omitted, the next statement in the subsequent case label gets executed. Because the break statement is optional, its omission produces no compiler warnings. If this behavior is unintentional, it can lead to undesirable control flows.
Noncompliant Code Example
In this noncompliant code example, the case wherein the card
is 11
does not have a break
statement. Thus, the statements for card = 12
are also executed when card = 11
.
int card = 11; switch (card) { /* ... */ case 11: System.out.println("Jack"); case 12: System.out.println("Queen"); break; case 13: System.out.println("King"); break; default: System.out.println("Invalid Card"); break; }
Compliant Solution
In this compliant solution, each case (including the default) is terminated by a break
statement.
int card = 11; switch (card) { /* ... */ case 11: System.out.println("Jack"); break; case 12: System.out.println("Queen"); break; case 13: System.out.println("King"); break; default: System.out.println("Invalid Card"); break; }
Exceptions
EX1: The last label in a switch statement requires no break. The break statement serves to skip to the end of the switch block, so control flow will continue to statements following the switch block with or without it. Conventionally, the last label is the default label.
EX2: In some cases, where control flow is intended to execute the same code for multiple cases, it is permissible to omit the break statement. However, these instances must be explicitly documented.
int card=11; int value; /* Case 11,12,13 fall through to the same case */ switch (card) { /* MSC13-J:EX2: these three cases are treated identically */ case 11: case 12: case 13: value=10; break; default: /* Handle Error Condition */ }
EX3: A case needs no break
statement if its last statement is a return
or throw
.
Risk Assessment
Failure to include break statements leads to unexpected control flow.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC13-J |
medium |
unlikely |
low |
P6 |
L2 |
Other Languages
This rule appears in the C Secure Coding Standard as MSC17-C. Finish every set of statements associated with a case label with a break statement.
This rule appears in the C++ Secure Coding Standard as MSC18-CPP. Finish every set of statements associated with a case label with a break statement.
References
[[JLS 05]] Section 14.11 The switch Statement