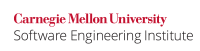
Java language enumeration types have an ordinal()
method, which returns the numerical position of each enumeration constant in its class declaration.
Section 8.9, "Enums" of the Java Language Specification [[JLS 2005]] does not specify the use of
ordinal()
in programs. However, Using the ordinal()
method to derive the value associated with an enum constant is error prone and should be avoided.
According to the Java API [[API 2006]], ordinal()
is defined as
|
Returns the ordinal of the enumeration constant (its position in its enum declaration, where the initial constant is assigned an ordinal of zero). Most programmers will have no use for this method. It is designed for use by sophisticated enum-based data structures, such as |
Noncompliant Code Example
This noncompliant code example declares enum Hydrocarbon
and uses its ordinal()
method to provide the result of the getNumberOfCarbons()
method.
enum HydroCarbon { METHANE, ETHANE, PROPANE, BUTANE, PENTANE, HEXANE, HEPTANE, OCTANE, NONANE, DECANE; public int getNumberOfCarbons() { return ordinal() + 1; } } public class TestHC { public static void main(String args[]) { /* ... */ HydroCarbon hc = HydroCarbon.HEXANE; int index = hc.getNumberOfCarbons(); int noHyd = NumberOfHydrogen[index]; // Can cause ArrayIndexOutOfBoundsException } }
While this noncompliant code example works, its maintenance is susceptible to vulnerabilities. If the enum constants were reordered, the getNumberOfCarbon()
method would return incorrect values. Also, BENZENE
— which also has 6 carbons — cannot be added without violating the current enum design.
Compliant Solution
In this compliant solution, enum constants are explicitly associated with the corresponding integer values.
enum HydroCarbon { METHANE(1), ETHANE(2), PROPANE(3), BUTANE(4), PENTANE(5), HEXANE(6), BENZENE(6), HEPTANE(7), OCTANE(8), NONANE(9), DECANE(10); private final int numberOfCarbons; Hydrocarbon(int carbons) { this.numberOfCarbons = carbons; } public int getNumberOfCarbons() { return numberOfCarbons; } }
Risk Assessment
Use of ordinals to derive integer values reduces the program's maintainability and can lead to errors in the program.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL11-J |
low |
probable |
medium |
P4 |
L3 |
Related Guidelines
C Secure Coding Standard: INT09-C. Ensure enumeration constants map to unique values
C++ Secure Coding Standard: INT09-CPP. Ensure enumeration constants map to unique values
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[JLS 2005]] Section 8.9, "Enums"
[[API 2006]] Enum
DCL10-J. Ensure proper initialization by declaring class and instance variables final 03. Declarations and Initialization (DCL) 04. Expressions (EXP)