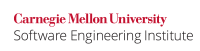
The enhanced for statement introduced in Java 1.5 (a.k.a. the for-each idiom) is primarily used for iterating over collections of objects. Unlike the original for statement, assignments to the loop variable fail to affect the loop's iteration order over the underlying set of objects. Thus, assignments to the loop variable can have an effect other than what is intended by the developer. This provides yet another reason to avoid assigning to the loop variable in a for loop.
As detailed in the Java Language Specification [[JLS 2005]], Section 14.14.2, "The Enhanced For Statement"
an enhanced for statement of the form
for (ObjType obj : someIterableItem) { // ... }is equivalent to a standard for loop of the form
for (Iterator myIterator = someIterableItem.iterator(); iterator.hasNext();) { ObjType obj = myIterator.next(); // ... }
Consequently, an assignment to the loop variable is equivalent to modifying a variable local to the loop body whose initial value is the object referenced by the loop iterator. This modification is not necessarily erroneous, but it can obscure the loop functionality or indicate a misunderstanding of the underlying implementation of the enhanced for statement.
Declare all enhanced for statement loop variables to be final. The final declaration causes Java compilers to flag and reject any assignments made to the loop variable.
Noncompliant Code Example
This noncompliant code example attempts to process a collection of objects using an enhanced for loop. It further intends to skip processing one item in the collection.
Collection<ProcessObj> processThese = // ... for (ProcessObj processMe: processThese) { if (someCondition) { // found the item to skip someCondition = false; processMe = processMe.getNext(); // attempt to skip to next item } processMe.doTheProcessing(); // process the object }
The attempt to skip to the next item is "successful" in the sense that the assignment succeeds and the value of processMe
is updated. Unlike an original for loop, however, the assignment leaves the overall iteration order of the loop unchanged. Thus, the object following the skipped object is processed twice; this is unlikely to be what the programmer intended.
Note that if processMe
were declared final, a compiler error would result at the attempted assignment.
Compliant Solution
This compliant solution correctly processes the objects in the collection at most once.
Collection<ProcessObj> processThese = // ... for (final ProcessObj processMe: processThese) { if (someCondition) { // found the item to skip someCondition = false; continue; // skip by continuing to next iteration } processMe.doTheProcessing(); // process the object }
Risk Assessment
Assignments to the loop variable of an enhanced for loop (for-each idiom) fail to affect the overall iteration order, lead to programmer confusion, and can leave data in a fragile or inconsistent state.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL05-J |
low |
unlikely |
low |
P3 |
L3 |
Automated Detection
Easily enforced with static analysis.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[JLS 2005]] Section 14.14.2 "The enhanced for statement"
DCL04-J. Do not apply public final to constants whose value might change Declarations and Initialization (DCL) DCL06-J. Use 'L', not 'l', to indicate that an integer literal is of type long