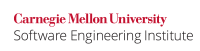
Avoid performing bitwise and arithmetic operations on the same data. In particular, bitwise operations are frequently performed on arithmetic values as a form of premature optimization. Bitwise operators include the unary operator ~ and the binary operators <<
, >>
, &
, ^, and |
. Although such operations are valid and will compile, they can reduce code readability. Declaring a variable as containing a numeric value or a bitmap makes the programmer's intentions clearer and the code more maintainable.
Left- and right-shift operators are often employed to multiply or divide a number by a power of 2. This compromises code readability and portability for the sake of often-illusory speed gains. The JVM will usually make such optimizations automatically, and unlike a programmer, the JVM can optimize for the implementation details of the current platform.
Noncompliant Code Example (Left Shift)
In this noncompliant code example, both bit manipulation and arithmetic manipulation are performed on the integer x
. The result is a (prematurely) optimized statement that assigns 5x + 1
to x
for implementations, where integers are represented as two's complement values.
int x = 50; x += (x << 2) + 1;
Compliant Solution (Left Shift)
In this compliant solution, the assignment statement is modified to reflect the arithmetic nature of x
, resulting in a clearer indication of the programmer's intentions.
int x = 50; x = 5 * x + 1;
A reviewer may now recognize that the operation should also be checked for overflow. This might not have been apparent in the original, noncompliant code example. For more information, see INT00-J. Ensure that integer operations do not result in overflow.
Noncompliant Code Example (Right Shift)
In this noncompliant code example, the programmer prematurely optimizes code by replacing a division with a right shift.
int x = -50; x = x + 2; x >>= 2;
Although this code will perform the division correctly, it is less readable and maintainable than actually making the division explicit.
Compliant Solution (Right Shift)
In this compliant solution, the right shift is replaced by division.
int x = -50; x += 2 x /= 4;
Exceptions
INT03-EX1: Bitwise operations may be used to construct constant expressions.
int limit = 1 << 17 - 1; // 2^17 - 1 = 131071
int03-EX2: Data that is normally treated arithmetically may be treated with bitwise operations for the purpose of serialization or deserialization. This is often required for reading or writing the data from a file or network socket, It may also be used when reading or writing the data from a tightly packed data structure of bytes.
int value = /* interesting value */ Byte bytes[] = new Byte[4]; for (int i = 0; i < bytes.length; i++) { bytes[i] = value >> (i*8) & 0xFF; } /* bytes[] now has same bit representation as value */
Risk Assessment
Performing bit manipulation and arithmetic operations on the same variable obscures the programmer's intentions and reduces readability. This in turn makes it more difficult for a security auditor or maintainer to determine which checks must be performed to eliminate security flaws and ensure data integrity.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
NUM01-J |
medium |
unlikely |
medium |
P4 |
L3 |
Related Guidelines
CERT C Secure Coding Standard: INT14-C. Avoid performing bitwise and arithmetic operations on the same data
CERT C++ Secure Coding Standard: INT14-CPP. Avoid performing bitwise and arithmetic operations on the same data
Bibliography
[[Steele 1977]]
INT13-C. Use bitwise operators only on unsigned operands 04. Integers (INT) INT15-C. Use intmax_t or uintmax_t for formatted IO on programmer-defined integer types