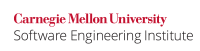
Static shared data should not be protected using instance locks because these are ineffective when two or more instances of the class are created. Consequently, the shared state is not safe for concurrent access unless a static lock object is used. If the class can interact with untrusted code, the lock must also be private and final, as per CON07-J. Use private final lock objects to synchronize classes that may interact with untrusted code.
Noncompliant Code Example (Non-static Lock Object for Static Data)
This noncompliant code example uses a non-static lock object to guard access to a static field counter
. If two Runnable
tasks are started, they will create two instances of the lock object and lock on each separately.
public final class CountBoxes implements Runnable { private static volatile int counter; // ... private final Object lock = new Object(); public void run() { synchronized(lock) { counter++; // ... } } public static void main(String[] args) { for(int i = 0; i < 2; i++) { new Thread(new CountBoxes()).start(); } } }
This does not prevent either thread from observing an inconsistent value of counter
because the increment operation on volatile fields is not atomic in the absence of proper synchronization (see CON02-J. Ensure that compound operations on shared variables are atomic).
Noncompliant Code Example (Method Synchronization for Static Data)
This noncompliant code example uses method synchronization to protect access to a static class field counter
.
public final class CountBoxes implements Runnable { private static volatile int counter; // ... public synchronized void run() { counter++; // ... } // ... }
In this case, the intrinsic lock is associated with each instance of the class and not with the class itself. Consequently, threads constructed using different Runnable
instances may observe inconsistent values of the counter
.
Compliant Solution (Static Lock Object)
This compliant solution declares the lock object as static and consequently, ensures the atomicity of the increment operation.
public class CountBoxes implements Runnable { private static int counter; // ... private static final Object lock = new Object(); public void run() { synchronized(lock) { counter++; // ... } // ... }
There is no need to declare the counter
variable volatile when synchronization is used.
Risk Assessment
Using an instance lock to protect static shared data can result in non-deterministic behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON13- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
The following table summarizes the examples flagged as violations by SureLogic Flashlight:
Noncompliant Code Example |
Flagged |
Message |
---|---|---|
non-static lock object for |
No |
No obvious issues |
method synchronization for |
No |
No obvious issues |
The following table summarizes the examples flagged as violations by SureLogic JSure:
Noncompliant Code Example |
Flagged |
Message |
---|---|---|
non-static lock object for |
Yes |
modeling problem: static region "counter" should be protected by a static field |
method synchronization for |
Yes |
modeling problem: static region "counter" should be protected by a static field |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]]
Issue Tracking
Review List
CON33-J. Document thread-safety and use annotations where applicable 11. Concurrency (CON) CON10-J. Do not synchronize on the intrinsic locks of high-level concurrency objects