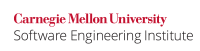
The char
type is the only unsigned primitive type in Java. As a result, a signed value cannot be stored and retrieved successfully from a variable of type char
.
In particular, comparing a value of type char
with -1 will never yield true
. However, because the method read()
returns -1 to indicate EOF
, it is tempting to compare the character returned by read()
with -1. This is a common error [[Pugh 08]].
Noncompliant Code Example
In this noncompliant code, the value of type int
returned by the read()
method is cast directly to a value of type char
which is compared with -1 to try to detect EOF
. This conversion leaves the value of c
as 0xffff (Character.MAX_VALUE
) instead of -1. Hence, this test never evaluates to true
.
char c; while ((c=(char)in.read())!= -1) { ... }
Compliant Solution
Always use a signed type of sufficient size to store signed data. To be compliant, use a value of type int
to check for EOF
while reading in data. If the value of type int
returned by read()
is not -1, then it can be safely cast to a value of type char
.
int c; while ((c=in.read())!= -1) {ch = (char)c; ... }
Risk Assessment
Storing signed data in a variable of the unsigned type char
can lead to misinterpreted data and possibly to memory leaks. Furthermore, comparing a value of type char
with -1 never evaluates to true
. This error could lead to a denial-of-service attack.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT35- J |
low |
unlikely |
low |
P3 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Secure Coding Standard as FIO34-C. Use int to capture the return value of character IO functions.
This rule appears in the C++ Secure Coding Standard as FIO34-CPP. Use int to capture the return value of character IO functions.
References
[[API 06]] Class InputStream
[[JLS 05]] 4.2 Primitive Types and Values
[[Pugh 08]] "Waiting for the end"