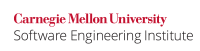
The Java programming language supports overloading methods, and Java can distinguish between methods with different method signatures. This means that, with some qualifications, methods within a class can have the same name if they have different parameter lists. In method overloading the choice of which method to invoke is determined at compile time. Even if the runtime type differs for each invocation, in overloading, the method invocations depend on the type of the object at compile time.
Overloaded methods should be used sparingly, as they can make code much less readable [[Tutorials 2010]]. Do not introduce ambiguity while overloading, for similar reasons [[Bloch 2008]].
Noncompliant Code Example
This noncompliant code example attempts to use the overloaded display method to perform different actions depending on whether the method is passed an ArrayList<Integer>
or a LinkedList<String>
.
public class Overloader { private static String display(ArrayList<Integer> a) { return "ArrayList"; } private static String display(LinkedList<String> l) { return "LinkedList"; } private static String display(List<?> l) { return "List is not recognized"; } public static void main(String[] args) { // Array of lists List<?>[] invokeAll = new List<?>[] {new ArrayList<Integer>(), new LinkedList<String>(), new Vector<Integer>()}; for (List<?> i : invokeAll) { System.out.println(display(i)); } } }
At compile time, the type of the object array is List
. The expected output is ArrayList
, LinkedList
and List is not recognized
(java.util.Vector
does not inherit from java.util.List
). However, in all three instances List is not recognized
is displayed. This happens because in overloading, the method invocations are not affected by the runtime types but only the compile time type (List
). It is dangerous to implement overloading to tally with overriding, more so, because the latter is characterized by inheritance unlike the former [[Bloch 2008]].
Compliant Solution
This compliant solution uses a single display
method and instanceof
to distinguish between different types. As expected, the output is ArrayList
, LinkedList
, List is not recognized
.
class Overloader { public class Overloader { private static String display(List<?> l) { return ( l instanceof ArrayList ? "Arraylist" : (l instanceof LinkedList ? "LinkedList" : "List is not recognized") ); } public static void main(String[] args) { List<?>[] invokeAll = new List<?>[] {new ArrayList<Integer>(), new LinkedList<String>(), new Vector<Integer>()}; for (List<?> i : invokeAll) { System.out.println(display(i)); } } }
Risk Assessment
Ambiguous uses of overloading can lead to unexpected results.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MET05-J |
low |
unlikely |
high |
P1 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[API 2006]] Interface Collection
[[Bloch 2008]] Item 41: Use overloading judiciously
[[Tutorials 2010]] Defining Methods
MET04-J. Ensure that constructors do not call overridable methods 16. Methods (MET) MET06-J. Do not call overridable methods from a privileged block