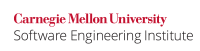
Problems may arise if defensive copies of untrusted method parameters are made and security decisions are based on these copies. An attacker can sufficiently bypass security checks under such circumstances. An example of an untrusted method argument is an object instance whose class provides a clone()
method and the class itself is nonfinal.
Noncompliant Code Example
This noncompliant code example accepts an untrusted parameter and creates a copy using the clone()
method. This is insecure because a copy of the attacker's class is created instead of the system class. Input validation routines may not work as expected when the attacker overrides the getTime()
method so that it passes validation when called for the first time, but mutates when it is used a second time. Here, the validateValue()
method is required to protect insertion of time data prior to some known time but fails to achieve this purpose.
private Boolean validateValue(long time) { // Perform validation return true; // If the time is valid } private void storeDateinDB(java.util.Date date) throws SQLException { final java.util.Date copy = (java.util.Date)date.clone(); validateValue(copy.getTime()); Connection con = DriverManager.getConnection("jdbc:microsoft:sqlserver://<HOST>:1433","<UID>","<PWD>"); PreparedStatement pstmt = con.prepareStatement("UPDATE ACCESSDB SET TIME = ?"); pstmt.setLong(1, copy.getTime()); // ... }
The attacker can override the getTime()
method as shown below.
public class MaliciousDate extends java.util.Date { private static int count = 0; @Override public long getTime() { java.util.Date d = new java.util.Date(); return (count++ == 1) ? d.getTime() : d.getTime() - 1000; } }
Compliant Solution
This compiant solution creates a new java.util.Date
object which is subsequently used for access control checks and insertion into the database.
private void storeDateinDB(java.util.Date date) throws SQLException { final java.util.Date copy = new java.util.Date(date.getTime()); validateValue(copy.getTime()); Connection con = DriverManager.getConnection("jdbc:microsoft:sqlserver://<HOST>:1433","<UID>","<PWD>"); PreparedStatement pstmt = con.prepareStatement("UPDATE ACCESSDB SET TIME = ?"); pstmt.setLong(1, copy.getTime()); // ... }
Risk Assessment
Using the clone()
method to copy untrusted parameters can result in the execution of arbitrary code.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MET08-J |
high |
likely |
low |
P27 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[Sterbenz 2006]]
MET07-J. Do not invoke overridable methods on the clone under construction 16. Methods (MET) MET09-J. Always provide feedback about the resulting value of a method