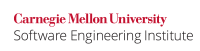
The conditional operator ?:
uses the boolean
value of its first operand to decide which of the other two expressions will be evaluated (see JLS, Section 15.25, "Conditional Operator ? :
".)
The general form of a Java conditional expression is operand1 ? operand2 : operand3
.
- If the value of the first operand (
operand1
) istrue
, then the second operand expression (operand2
) is chosen. - If the value of the first operand is
false
, then the third operand expression (operand3
) is chosen.
The conditional operator is syntactically right-associative; for example, a?b:c?d:e?f:g
is equivalent to a?b:(c?d:(e?f:g))
.
The JLS-defined rules for determining the type of the result of a conditional expression (tabulated below) are complicated; programmers could be surprised by the type conversions required for expressions they have written.
Result type determination begins from the top of the table; the compiler applies the first matching rule. The "Operand 2" and "Operand 3" columns refer to operand2
and operand3
(from the above definition), respectively. In the table, constant int
refers to constant expressions of type int
(such as '0' or variables declared final
).
Operand 2 |
Operand 3 |
Resultant type |
---|---|---|
type T |
type T |
type T |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
other numeric |
other numeric |
promoted type of the 2nd and 3rd operands |
T1 = boxing conversion (S1) |
T2 = boxing conversion(S2) |
apply capture conversion to lub(T1,T2) |
See JLS, Section 5.1.7, "Boxing Conversion"; JLS, Section 5.1.10, "Capture Conversion"; and JLS, Section 15.12.2.7, "Inferring Type Arguments Based on Actual Arguments" for additional information on the final table entry.
The complexity of the rules that determine the result type of a conditional expression can lead to unintended type conversions. Consequently, the second and third operands of each conditional expression should have identical types. This recommendation also applies to boxed primitives.
Noncompliant Code Example
In this noncompliant code example, the programmer expects that both print statements will print the value of alpha
as a char
— A
. The first print statement indeed prints A
, because the compiler applies the eighth rule in the table above to determine that the second and third operands of the conditional expression are, or are converted to, type char
. However, the second print statement prints 65
— the value of alpha
as an int
. The first matching rule from the table above is the tenth rule; consequently, the compiler promotes the value of alpha
to type int
.
public class Expr { public static void main(String[] args) { char alpha = 'A'; int i = 0; ... // other code. Value of i may change boolean trueExp = ...; // some expression that evaluates to true System.out.print(trueExp ? alpha : 0); // prints A System.out.print(trueExp ? alpha : i); // prints 65 } }
Compliant Solution
This compliant solution uses identical types for the second and third operands of each conditional expression; the explicit casts specify the type expected by the programmer.
public class Expr { public static void main(String[] args) { char alpha = 'A'; int i = 0; boolean trueExp = ...; // some expression that evaluates to true System.out.print(trueExp ? alpha : ((char) 0)); // prints A // Intentional truncation of i System.out.print(trueExp ? alpha : ((char) i)); // prints A } }
Note that the explicit cast in the first conditional expression is redundant; that is, the value printed remains identical whether the cast is present or absent. Nevertheless, use of the redundant cast is good practice; it serves as an explicit indication of the programmer's intent, and consequently improves maintainability. When the value of i
in the second conditional expression falls outside the range that can be represented as a char
, the explicit cast will truncate its value. Although this appears to violate guideline EXP13-J. Do not cast numeric types to narrower types without a range check, in this case the truncation is both intended and also documented as intended. Consequently, this code falls under exception EXP13-EX1 and conforms with the guideline.
Noncompliant Code Example
This noncompliant example prints {{65}}â”the ASCII equivalent of A
, instead of the expected A
, because the second operand (alpha
) must be promoted to type int
. The numeric promotion occurs because the value of the third operand (the constant expression '123456') is too large to be represented as a char
.
public class Expr { public static void main(String[] args) { char alpha = 'A'; System.out.print(true ? alpha : 123456); } }
Compliant Solution
The compliant solution explicitly states the intended result type by casting alpha
to type int
. Casting 123456
to type char
would ensure that both operands of the conditional expression have the same type, resulting in A
being printed. However, it would result in data loss when an integer larger than Character.MAX_VALUE
is downcast to type char
. This compliant solution avoids potential truncation by casting alpha
to type int
, the wider of the operand types.
public class Expr { public static void main(String[] args) { char alpha = 'A'; // Cast alpha as an int to explicitly state that the type of the // conditional expression should be int. System.out.print(true ? ((int) alpha) : 123456); } }
Noncompliant Code Example
This noncompliant code example prints 65
instead of A
. The third operand is a variable of type int
, so the second operand (alpha
) must be converted to type int
.
public class Expr { public static void main(String[] args) { char alpha = 'A'; int i = 0; System.out.print(true ? alpha : i); } }
Compliant Solution
This compliant solution declares i
as type char
, ensuring that the second and third operands of the conditional expression have the same type.
public class Expr { public static void main(String[] args) { char alpha = 'A'; char i = 0; //declare as char System.out.print(true ? alpha : i); } }
Noncompliant Code Example
This noncompliant code example uses boxed and unboxed primitives of different types in the conditional expression. Consequently, the Integer
object is auto-unboxed to its primitive type int
and then converted to the primitive type float
, resulting in loss of precision [[Findbugs 2008]].
public class Expr { public static void main(String[] args) { Integer i = Integer.MAX_VALUE; float f = 0; System.out.print(true ? i : f); } }
Compliant Solution
This compliant solution declares both the operands as Integer
.
public class Expr { public static void main(String[] args) { Integer i = Integer.MAX_VALUE; Integer f = 0; //declare as Integer System.out.print(true ? i : f); } }
Risk Assessment
When the second and third operands of a conditional expression have different types, they can be subject to type conversions that were not anticipated by the programmer.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP14-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
Automated detection of condition expressions whose second and third operands are of different types is straightforward.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[Bloch 2005]] Puzzle 8: Dos Equis
[[Findbugs 2008]] "Bx: Primitive value is unboxed and coerced for ternary operator"
[[JLS 2005]] Section 15.25, "Conditional Operator
? :
"