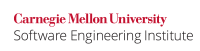
?Java supports the use of various types of literals, such as integers (5, 2), floating point numbers (2.5, 6.022e+23), characters ('a', '\n'), booleans ('true', 'false'), and strings ("Hello\n"). Extensive use of literals within a program can lead to two problems: first, the meaning of the literal is often obscured or unclear from the context (from which they derive the name "magic numbers"), and second, changing a frequently-used literal requires the entire program source code to be searched for occurances of that literal, creating possible error sources if some of the occurances of the literal are overlooked.
A solution to this problem is to declare meaningfully-named constants at the start of the program whose values are set equal to the desired literals, and to refrence these constants rather than the literals themselves throughout the program. The advantages to this approach are that the constant's name can indicate the meaning or intended use of the constant, and should the constant need to be changed, this can be accomplished simply by editing the constant declaration without having to search the code for all uses of it.
final
The final
keyword in Java is used to declare constants. Its effect is to render the affected variable immutable. Attempting to change the value of a final
-qualified variable results in a compile-time error.
The following code fragment demonstrates its use:
final int SIZE=25;
This code declares the value SIZE to be of type int and to have the immutable value 25. This constant can subsequently be used whenever the value 25 would be needed.
Noncompliant Code Example
The following noncompliant code calculates various dimensions of a sphere, given its radius.
double area(double radius){ return 12.56*radius*radius;} double volume(double radius){ return 4.19*radius*radius*radius;} double greatCircleCircumference(double radius){ return 6.28*radius;}
The methods use the seemingly-random literals 12.56, 4.19, and 6.28 to represent various scaling factors used to calculate these dimensions. Someone reading this code would have no idea how they were generated or what they meant, and would therefore be unable to understand the function of this code.
Noncompliant Code Example
The following noncompliant code attempts to avoid the above issues by explicitly calculating the required constants
double area(double radius){ return 4.0*3.14*radius*radius;} double volume(double radius){ return 4.0/3.0*3.14*radius*radius*radius;} double greatCircleCircumference(double radius){ return 2*3.14*radius;}
The code uses the literal "3.14" to represent the value pi. Although this removes some of the ambiguity from the literals, it complicates code maintenance. If the programmer were to decide that a more-precise value of pi was needed, he would need to find all occurances of "3.14" in the code and replace them.
Compliant Solution
In this compliant solution, a constant PI is first declared and set equal to 3.14, and is thereafter referenced in the code whenever the value pi is needed.
final int PI = 3.14; double area(double radius){ return 4.0*PI*radius*radius;} double volume(double radius){ return 4.0/3.0*PI*radius*radius*radius;} double greatCircleCircumference(double radius){ return 2*PI*radius;}
This both clarifies the code and allows easy editing, for if a different value for pi is required, the programmer can simply redefine the constant.