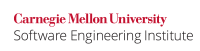
The final
keyword identifies constant values. That is, final
indicates fields whose value cannot change during an invocation of a program.
The JLS allows implementations to insert the value of public final fields inline in any compilation unit that reads the field. Consequently, if the declaring class is edited such that the new version gives a different value for the field, compilation units that read the public final field may still see the old value until they are themselves re-compiled.
A related error can arise when a programmer declares a static final
reference to a mutable object; see guideline OBJ01-J. Do not assume that declaring a reference to be final causes the referenced object to be immutable for additional information.
Noncompliant Code Example
In this noncompliant code example, class Foo
declares a field whose value represents the version of the software. The field is subsequently accessed by class Bar
, which lives in a separate compilation unit.
Foo.java:
class Foo { static public final int VERSION = 1; // ... }
Bar.java:
class Bar { public static void main(String[] args) { printf("You are using version " + Foo.VERSION); } }
When compiled and run, the software correctly prints:
You are using version 1
However, a subtle flaw is possible in the future. Suppose a developer updates the version number by modifying Foo.java, changing the value of VERSION
to be 2. The developer then recompiles Foo.java, but fails to recompile Bar.java. Now the software incorrectly prints:
You are using version 1
because Bar.java still thinks that Foo.VERSION
is 1.
Although recompiling Bar.java will solve this problem, a better solution is available.
Compliant Solution
According to the Java Language Specification [[JLS 2005]], Section 13.4.9, "final
Fields and Constants"
Other than for true mathematical constants, we recommend that source code make very sparing use of class variables that are declared
static
andfinal
. If the read-only nature offinal
is required, a better choice is to declare aprivate static
variable and a suitable accessor method to get its value.
Thus a compliant solution would be:
Foo.java:
class Foo { static private final int version = 1; static public final String getVersion() { return version; } // ... }
Bar.java:
class Bar { public static void main(String[] args) { printf("You are using version " + Foo.getVersion()); } }
The private version value can therefore not be copied into the Bar class when it is compiled, thus preventing the bug. Note that most JITs are capable of inlining the getVersion()
method at runtime; consequently there is little or no performance penalty incurred.
Exceptions
DCL04-EX1: According to the Java Language Specification [[JLS 2005]], Section 9.3 "Field (Constant) Declarations," "Every field declaration in the body of an interface is implicitly public
, static
, and final
. It is permitted to redundantly specify any or all of these modifiers for such fields."
DCL04-EX2: Constants declared using the enum
type may violate this guideline.
DCL04-EX3: Constants that never change their values throughout the lifetime of the software may indeed be declared final. For instance, the JLS recommends that mathematical constants be declared final.
Risk Assessment
Failing to declare mathematical constants static
and final
can lead to thread safety issues as well as to inconsistent behavior.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL04-J |
low |
probable |
medium |
P2 |
L3 |
Automated Detection
Static checking of this guideline is not feasible in the general case.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Related Guidelines
C Secure Coding Standard: DCL00-C. Const-qualify immutable objects
Bibliography
[[JLS 2005]] "13.4.9 final Fields and Constants", "9.3 Field (Constant) Declarations", "4.12.4 final Variables", "8.3.1.1 static Fields"
DCL03-J. Properly encode relationships in constant definitions 03. Declarations and Initialization (DCL) DCL05-J. Do not attempt to assign to the loop variable in an enhanced for loop