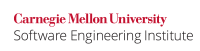
In the presence of a security manager and a restrictive system-wide security policy, untrusted code is prohibited from performing privileged operations. For example, instantiation of sensitive classes such as java.lang.ClassLoader
is prohibited in the context of a web browser. At the same time, it is critical to ensure that untrusted code does not indirectly use the privileges of trusted code to perform privileged operations. Most APIs install security manager checks to prevent this, however, some do not. These APIs are tabulated below.
APIs |
---|
|
|
|
|
|
|
|
|
|
|
These APIs perform tasks using the immediate caller's class loader. They can be exploited if (1) They are invoked indirectly by untrusted code and/or (2) They accept tainted inputs from untrusted code.
Classes that have the same defining class loader exist in the same namespace but may have different privileges, depending on the security policy. Security vulnerabilities can arise if the trusted code coexists with untrusted code, and both have the same defining class loader. This is because untrusted code can freely access members of the trusted code depending on their accessibility. If the trusted code uses any of the tabulated APIs, no security manager checks are carried out.
Sometimes untrusted code is loaded by a class loader instance that is different from the one used to load the trusted code. Security vulnerabilities can arise if the untrusted code's class loader delegates to the trusted code's class loader. In the absence of such a delegation relationship, the class loaders ensure namespace separation and disallow untrusted code from observing members or invoking methods belonging to the trusted code.
Consider for example, untrusted code that is attempting to load a privileged class. Its class loader is permitted to delegate the class loading to the trusted class's class loader. This is a problem as untrusted code's class loader may not have the permission to load the particular class. Also, if the trusted code accepts tainted inputs, malicious classes may be loaded on behalf of untrusted code.
Noncompliant Code Example
The untrustedCode()
method of class Untrusted
invokes the loadLib()
method of class NativeCode
in this noncompliant code example. This is insecure as the library is loaded on behalf of untrusted code. In essence, the untrusted code's class loader may be able to indirectly load the intended library even if it does not have sufficient permissions.
class NativeCode { public native void loadLib(); static { try { System.loadLibrary("/com/foo/MyLib.so"); }catch(UnsatisfiedLinkError e) { e.getMessage(); } } } class Untrusted { public static void untrustedCode() { new NativeCode().loadLib(); } }
Sometimes, a call to System.loadLibrary()
is embedded in a doPrivileged
block, as shown below. An unprivileged caller can maliciously invoke this piece of code using the same technique because the doPrivileged
block allows security manager checks to be forgone for other callers on the execution chain.
AccessController.doPrivileged(new PrivilegedAction() { public Object run() { System.loadLibrary("awt"); return null; } });
Non-native library code can also be susceptible to related security flaws. Loading a non-native safe library, by itself may not expose a vulnerability but after loading an unsafe library, an attacker can easily exploit it if it contains other vulnerabilities. Moreover, non-native libraries often use doPrivileged
blocks, making them lucrative targets.
Compliant Solution
Ensure that untrusted code cannot invoke the affected APIs directly or indirectly (that is, via a call to an invoking method). In this case, the loadLib()
method must be declared private
so that it is only available to a more restrictive method within the class. The restrictive method can ensure that the caller has sufficient permissions to load the library.
private final native void loadLib();
Noncompliant Code Example
Accepting tainted inputs from untrusted code can further exacerbate the issue. The single argument Class.forname()
method is another example of an API that uses its immediate caller's class loader to load a desired class. Untrusted code can indirectly misuse this API to manufacture classes with the same privileges as those of the immediate caller.
// className may be the name of a privileged or even a malicious class Class c = Class.forName(className);
Compliant Solution
Limit the visibility of the method that uses this API. Do not operate on tainted inputs. This compliant solution hardcodes the class's name.
Class c = Class.forName("Foo"); // Explicitly hardcode
Noncompliant Code Example
This noncompliant code example returns an instance of java.sql.Connection
from trusted to untrusted code. The untrusted code that does not have the permissions to create an SQL connection can bypass this restriction by directly using the acquired instance.
public Connection getConnection() { // ... return DriverManager.getConnection(url, username, password); }
Compliant Solution
Ensure that instances of objects created using the unsafe methods are not returned to untrusted code. Furthermore, it is preferable to reduce the accessibility of methods that perform sensitive operations and define wrapper methods that are accessible from untrusted code.
private void getConnection() { // ... conn = DriverManager.getConnection(url, username, password); // Do what is is required here itself; do not return the connection } public void DoDatabaseOperationWrapper() { // Perform any checks or validate input getConnection(); }
Exceptions
EX1: It is permissible to use APIs that do not use the immediate caller's class loader instance. For example, the three-argument java.lang.Class.forName()
method requires an explicit argument that specifies the class loader instance to use. Do not use the immediate caller's class loader as the third argument if instances must be returned to untrusted code.
public static Class forName(String name, boolean initialize, ClassLoader loader) /* explicitly specify the class loader to use */ throws ClassNotFoundException
Risk Assessment
Allowing untrusted code to carry out actions using the immediate caller's class loader may allow it to execute with the same privileges as the immediate caller.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SEC33- J |
high |
probable |
medium |
P12 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[SCG 07]] Guideline 6-3 Safely invoke standard APIs that perform tasks using the immediate caller's class loader instance
SEC17-J. Create and sign a SignedObject before creating a SealedObject 02. Platform Security (SEC) SEC03-J. Do not allow tainted variables in doPrivileged blocks