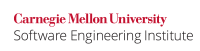
In the presence of a security manager, it is hard for malicious code to exploit Java's security model. For example, instantiating sensitive classes such as a ClassLoader
is prohibited in the context of a web browser. It is critical to ensure that untrusted code does not indirectly use the privileges of legit code. Failure to do so can leave the code vulnerable to privilege escalation attacks. This is because, classes loaded by the same class loader exist in the same namespace and will have identical privileges. Consider for example, an untrusted method calling a class method which loads classes using its own trusted class loader. This is a problem as untrusted code's class loader may not have the permission to load the particular class. Also, if the trusted code accepts tainted inputs, it is susceptible to exploits resulting from malicious classes getting loaded.
The APIs tabulated here perform tasks using the immediate caller's class loader. They can be exploited if (1) They are invoked indirectly by untrusted code and (2) They accept tainted inputs from untrusted code.
APIs |
---|
|
|
|
|
|
|
|
|
|
|
Noncompliant Code Example
The untrustedCode
method of class Untrusted
invokes loadLib
method of class NativeCode
in this noncompliant example. This is insecure as the library gets loaded on behalf of the untrusted code. In essence, the untrusted code's class loader may be able to load the intended library even if it does not have sufficient permissions.
class NativeCode { public native void loadLib(); static { try { System.loadLibrary("/com/foo/MyLib.so"); }catch(UnsatisfiedLinkError e) { e.getMessage(); } } } class Untrusted { public static void untrustedCode() { new NativeCode().loadLib(); } }
Sometimes, a call to System.loadLibrary
is embedded in a doPrivileged
block, as shown below. An unprivileged caller can maliciously invoke this piece of code using the same technique.
AccessController.doPrivileged(new PrivilegedAction() { public Object run() { System.loadLibrary("awt"); return null; } });
Non-native library code can also be susceptible to related security flaws. Loading a non-native safe library, by itself may not expose a vulnerability but after loading an unsafe library, an attacker can easily exploit it if it contains other vulnerabilities. Moreover, non-native libraries often make use of doPrivileged
blocks, making them a lucrative target.
Compliant Solution
Ensure that untrusted code cannot invoke the affected APIs directly or indirectly (that is, via a call to an invoking method). In this case, the loadLib()
method must be declared private
so that it is only available to a more restrictive method within the class. The restrictive method can ensure that the caller has sufficient permissions to load the library.
private final native void loadLib();
Noncompliant Code Example
Accepting tainted inputs from untrusted code can further exacerbate the issue. The single argument Class.forname()
method is another example of an API that uses its immediate caller's class loader to load a desired class. Untrusted code can indirectly misuse this API to manufacture classes with the same privileges as those of the immediate caller.
// className is Foo Class c = Class.forName(className);
Compliant Solution
Again, limit the visibility of the method that uses this API. Do not operate on tainted inputs. The preceding noncompliant code example can be fixed by hard-coding the class's name.
Class c = Class.forName("Foo"); // explicitly hardcode
Noncompliant Code Example
This noncompliant code example returns an instance of the immediate caller's class loader to any invoker. A malicious invoker can therefore, obtain the associated class loader using standard APIs such as java.lang.Class.getClassLoader()
. Once this is achieved, it is trivial to use Class.forname()
to load the malicious class from attacker space or exploit a trusted method that calls Class.newInstance()
on an arbitrarily supplied object. Class.newInstance()
does not throw any security exception when the class loader is either the same or the delegation ancestor of its immediate caller. (SEC02-J. Do not expose standard APIs that may bypass Security Manager checks to untrusted code)
private Class doLogic() { ClassLoader myLoader = new myClassLoader(); Class myClass = myLoader.loadClass("MyClass"); return myClass; // returns Class instance to untrusted code }
Compliant Solution
Always make sure that any internal Class
, ClassLoader
and Thread
instances are not returned to untrusted code.
private void doLogic() { ClassLoader myLoader = new myClassLoader(); Class myClass = myLoader.loadClass("MyClass"); // do what is is required here itself; do not return myClass }
Exceptions
EX1: It is permissible to use APIs that do not use the immediate caller's class loader instance. For example, the three-argument java.lang.Class.forName()
method requires an explicit argument that specifies the class loader instance to use.
public static Class forName(String name, boolean initialize, ClassLoader loader) // explicitly specify the class loader to use throws ClassNotFoundException
Risk Assessment
Allowing untrusted code to carry out actions using the immediate caller's class loader may allow it to execute with the same privileges as the immediate caller.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SEC03-J |
high |
probable |
medium |
P12 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[SCG 07]] Guideline 6-3 Safely invoke standard APIs that perform tasks using the immediate caller's class loader instance
SEC02-J. Do not expose standard APIs that may bypass Security Manager checks to untrusted code 01. Platform Security (SEC) SEC03-J. Do not allow tainted parameters while using APIs that perform access checks against the immediate caller