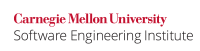
The conditional operator ?:
uses the boolean
value of one expression to decide which of the other two expressions should be evaluated [[JLS 05]].
The conditional operator is syntactically right-associative. For instance a?b:c?d:e?f:g
is equivalent to a?b:(c?d:(e?f:g))
.
Format:
ConditionalExpression:
ConditionalOrExpression
ConditionalOrExpression ? Expression : ConditionalExpression
- If the value of the first operand is
true
, then the second operand expression is chosen - If the value of the first operand is
false
, then the third operand expression is chosen
The rules that define the resultant type are given in the following table, where the first match, starting from the top, is used. In the table, *
refers to constant expressions of type int
(such as '0' or variables declared final
):
Operand 2 |
Operand 3 |
Resultant type |
---|---|---|
type T |
type T |
type T |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
other numeric |
other numeric |
promoted type of the 2nd and 3rd operands |
T1 = boxing conversion (S1) |
T2 = boxing conversion(S2) |
apply capture conversion to lub(T1,T2) |
Noncompliant Code Example
This noncompliant example prints A65
instead of AA
. The first print statement prints the value of alpha
as A
which is of the char
type. The third operand '0', is a constant expression of type int
and does not cause any numeric promotion. The second statement, however, prints 65
, the integer equivalent of A
. This is because of numeric promotion of the third operand alpha
to an int
which happens as a result of variable i
being an int
.
public class Expr { public static void main(String[] args) { char alpha = 'A'; int i = 0; System.out.print(true ? alpha : 0); System.out.print(false ? i : alpha); } }
Compliant Solution
This compliant solution recommends the use of the same types for the second and third operands of the conditional expressions. The clearer semantics help avoid confusion.
public class Expr { public static void main(String[] args) { char alpha = 'A'; char i = 0; //declare as char System.out.print(true ? alpha : 0); System.out.print(false ? i : alpha); } }
Exceptions
EXP00: It is permissible to use operands of different types when the offending type is declred final
. Consequently, it turns into a constant expression and numeric promotion does not occur.
public class Expr { public static void main(String[] args) { char alpha = 'A'; final int i = 0; System.out.print(true ? alpha : 0); System.out.print(false ? i : alpha); } }
Risk Assessment
If the types of the second and third operands in a conditional expression are not the same then the result of the conditional expression may be unexpected.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP00- J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Section 15.25, Conditional Operator ? :
[[Bloch 05]] Puzzle 8: Dos Equis
03. Expressions (EXP) 03. Expressions (EXP) EXP01-J. Ensure a null pointer is not dereferenced