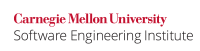
Java code that deals with input, for example Scanner
, often buffers its underlying InputStream
.
It is possible to create multiple wrappers around an InputStream
that buffer the input from that InputStream
. Such programs behave significantly differently depending on whether the InputStream
does or does not allow for read ahead. An adversary can exploit this difference in behavior by, for example, redirecting the InputStream
, which could lead to exploitable behavior.
Although the Java standard does not specifically mention this behavior, code compiled with javac
and run with the java
command exhibits this behavior on Java 1.5.0.
Do not create multiple wrappers that buffer their input on an InputStream
; create and use only one, either by passing it as an argument to the methods that need it or centralizing its use in a single place.
Noncompliant Code Example
This noncompliant code example creates multiple BufferedInputStreams
on System.in
. Although it will work when System.in
refers to a console, it crashes when System.in
has been redirected. Note that while this code uses a BufferedInputStream
to illustrate that any buffered wrapper is unsafe, it is also exploitable if a Scanner
is used instead.
import java.io.BufferedInputStream; import java.io.IOException; public final class InputLibrary{ public static char getChar()throws IOException { BufferedInputStream in = new BufferedInputStream(System.in); int input = in.read(); if(input==-1){ throw new IOException(); } return (char)input; } public static void main(String[] args)throws IOException { System.out.print("Enter first initial: "); char first=getChar(); System.out.println("Your first initial is "+first); System.out.print("Enter last initial: "); char last=getChar(); System.out.println("Your last initial is "+last); } }
Compliant Solution
Create and use only a single BufferedInputStream
on System.in
. This code example stores the BufferedInputStream
as a class variable so all methods can access it. However, if a program were to use this library in conjunction with other input from a user that also needs some buffered wrapper on System.in
, the library would need to be modified so that all code uses the same buffered wrapper instead of creating separate ones.
import java.io.BufferedInputStream; import java.io.IOException; public final class InputLibrary{ private static BufferedInputStream in = new BufferedInputStream(System.in); public static char getChar()throws IOException { int input = in.read(); if(input==-1){ throw new IOException(); } in.skip(1); //This line now necessary to go to the next line //in the previous example code deceptively worked without it return (char)input; } public static void main(String[] args)throws IOException { System.out.print("Enter first initial: "); char first=getChar(); System.out.println("Your first initial is "+first); System.out.print("Enter last initial: "); char last=getChar(); System.out.println("Your last initial is "+last); } }
Risk Assessment
Creating multiple buffered wrappers on an InputStream
can crash the program when the InputStream
is re-directed.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO39-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] class BufferedInputStream