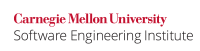
Thread-safety guarantees that no two threads can simultaneously access or modify some shared data. However, if two or more operations need to be performed safely, it becomes necessary to enforce atomicity. It is possible for two threads to read some shared value, independently perform operations on it and induce a race condition while storing the final result.
Programmers sometimes assume that using a thread-safe Collection
does not require explicit synchronization which is a misleading thought. It follows that using a thread-safe Collection
by itself does not ensure program correctness unless special care is taken to ensure that the client performs all related and independently atomic operations, as one atomic operation. For example, the standard thread-safe API may not provide a method to find a particular person's record in a Hashtable
and update the corresponding payroll information. In such cases, a custom thread-safe method must be designed and used. This guideline shows the need of such a method that performs a group of independently atomic operations as one atomic operation, and also suggests techniques for incorporating the method using a custom API.
This guideline applies to all uses of Collection
classes including the thread-safe Hashtable
class. Enumerations of the objects of a Collection
and iterators also require explicit synchronization on the Collection
object or any single lock object.
Noncompliant Code Example (synchronizedList)
This noncompliant code example comprises an ArrayList
collection which is non-thread-safe by default. There is, however, a way around this drawback. Most thread-unsafe classes have a synchronized thread-safe version, for example, Collections.synchronizedList
is a good substitute for ArrayList
and Collections.synchronizedMap
is a good alternative to HashMap
. The atomicity pitfall described in the coming lines, remains to be addressed even when the particular Collection
offers thread-safety benefits.
class RaceCollection { private List<InetAddress> ips = Collections.synchronizedList(new ArrayList<InetAddress>()); public void addIPAddress(InetAddress ia) { // Validate ips.add(ia); } public void doSomething() throws UnknownHostException { addIPAddress(InetAddress.getLocalHost()); InetAddress[] ia = (InetAddress[]) ips.toArray(new InetAddress[0]); System.out.println("Number of IPs: " + ia.length); } }
When the doSomething()
method is invoked on the same object from multiple threads, the output, consisting of varying array lengths, indicates a race condition between the threads. In other words, the statements that are responsible for adding an IP address and printing it out are not sequentially consistent. Also note that the operations within a thread's run()
method are non-atomic.
Noncompliant Code Example (Subclass)
This noncompliant code example extends the base class and synchronizes on the doSomething()
method which is required to be atomic.
class RaceCollectionSub extends RaceCollection { public synchronized void doSomething() throws UnknownHostException { addIPAddress(InetAddress.getLocalHost()); InetAddress[] ia = (InetAddress[]) ips.toArray(new InetAddress[0]); System.out.println("Number of IPs: " + ia.length); } }
However, this is not recommended because it goes against the spirit of limiting class extension ([OBJ05-J. Limit the extensibility of non-final classes and methods to only trusted subclasses]). Moreover, Goetz et al. [[Goetz 06]] cite other reasons:
Extension is more fragile than adding code directly to a class, because the implementation of the synchronization policy is now distributed over multiple, separately maintained source files. If the underlying class were to change its synchronization policy by choosing a different lock to guard its state variables, the subclass would subtly and silently break, because it no longer used the right lock to control concurrent access to the base class's state.
Moreover, when a wrapper such as Collections.synchronizedList()
is used (as shown in the previous noncompliant code example), it is unwieldy for a client to determine the type of the class (List
) that is being wrapped. Consequently, it is not directly possible to extend the class [[Goetz 06]].
Noncompliant Code Example (Method synchronization)
This noncompliant code example appears to use synchronization when defining the doSomething()
method, however, it acquires an intrinsic lock instead of the lock of the List
object. This means that another thread may modify the value of the List
instance when the doSomething()
method is executing.
class Helper { public List<InetAddress> ips = Collections.synchronizedList(new ArrayList<InetAddress>()); public synchronized void addIPAddress(InetAddress ia) { // Validate ips.add(ia); } public synchronized void doSomething() throws UnknownHostException { InetAddress[] ia; ia = (InetAddress[]) ips.toArray(new InetAddress[0]); System.out.println("Number of IPs: " + ia.length); } }
This noncompliant code example also violates OBJ00-J. Declare data members private.
Compliant Solution (Synchronized block)
To eliminate the race condition, ensure atomicity by using the underlying list's lock. This can be achieved by including all statements that use the array list within a synchronized block that locks on the list. This technique is also called client-side locking [[Goetz 06]].
public void addIPAddress(InetAddress ia) { synchronized(ips) { // Also synchronize this method // Validate ips.add(ia); } } public void doSomething() throws UnknownHostException { synchronized(ips) { addIPAddress(InetAddress.getLocalHost()); ia = (InetAddress[]) ips.toArray(new InetAddress[0]); System.out.println("Number of IPs: " + ia.length); } }
Goetz et al. [[Goetz 06]] caution against misuse of client-side locking:
If extending a class to add another atomic operation is fragile because it distributes the locking code for a class over multiple classes in an object hierarchy, client-side locking is even more fragile because it entails putting locking code for class C into classes that are totally unrelated to C. Exercise care when using client-side locking on classes that do not commit to their locking strategy.
Although expensive, CopyOnWriteArrayList
and CopyOnWriteArraySet
classes are sometimes used to create copies of the core Collection
so that iterators do not fail with a runtime exception when some data in the Collection
is modified. However, any updates to the Collection
are not immediately visible to other threads. Consequently, their use is limited to boosting performance in code where the writes are fewer (or non-existent) as compared to the reads [[JavaThreads 04]]. In all other cases they must be avoided.
Compliant Solution (Composition)
Composition offers more benefits as compared to the previous solution, although at the cost of a slight performance penalty (refer to OBJ07-J. Understand how a superclass can affect a subclass for details on how to implement composition).
class CompositeCollection { private List<InetAddress> ips; public CompositeCollection(List<InetAddress> list) { this.ips = list; } public synchronized void addIPAddress(InetAddress ia) { // Validate ips.add(ia); } public synchronized void doSomething() throws UnknownHostException { InetAddress[] ia; ia = (InetAddress[]) ips.toArray(new InetAddress[0]); System.out.println("Number of IPs: " + ia.length); } }
This approach allows the CompositeCollection
class to use its own intrinsic lock in a way that is completely independent of the lock of the underlying list class. Moreover, this permits the underlying collection to be thread-unsafe because the CompositeCollection
wrapper prevents direct accesses to its methods by exposing its own synchronized equivalents. This approach also provides consistent locking even when the underlying list is not thread-safe or when it changes its locking policy. [[Goetz 06]]
Noncompliant Code Example (synchronizedMap)
This noncompliant code example defines a thread-unsafe KeyedCounter
class. Even though the HashMap
field is synchronized, the overall increment
operation is not atomic. [[Lee 09]]
public class KeyedCounter { private Map<String, Integer> map = Collections.synchronizedMap(new HashMap<String, Integer>()); public void increment(String key) { Integer old = map.get(key); int value = (old == null) ? 1 : old.intValue() + 1; map.put(key, value); } public Integer getCount(String key) { return map.get(key); } }
Compliant Solution (Synchronized method)
This compliant solution declares the increment()
method as synchronized
to ensure atomicity [[Lee 09]].
public class KeyedCounter { private Map<String,Integer> map = new HashMap<String,Integer>(); public synchronized void increment(String key) { Integer old = map.get(key); int value = (old == null) ? 1 : old.intValue()+1; map.put(key, value); } public synchronized Integer getCount(String key) { return map.get(key); } }
Also, note that this would be a violation of a previously discussed noncompliant code example if the field map
were to refer to a Collections.synchronizedMap
object. This compliant solution uses the intrinsic lock of the class for all purposes.
Compliant Solution (ConcurrentHashMap)
The previous compliant solution does not scale very well because a class with several synchronized
methods is a potential bottleneck as far as acquiring locks is concerned and may further lead to contention or deadlock. The class ConcurrentHashMap
, through a more preferable approach, provides several utility methods to perform atomic operations and is used in this compliant solution [[Lee 09]]. According to Goetz et al. [[Goetz 06]] section 5.2.1. ConcurrentHashMap:
ConcurrentHashMap
, along with the other concurrent collections, further improve on the synchronized collection classes by providing iterators that do not throwConcurrentModificationException
, as a result eliminating the need to lock the collection during iteration. The iterators returned byConcurrentHashMap
are weakly consistent instead of fail-fast. A weakly consistent iterator can tolerate concurrent modification, traverses elements as they existed when the iterator was constructed, and may (but is not guaranteed to) reflect modifications to the collection after the construction of the iterator.
public class KeyedCounter { private final ConcurrentMap<String, AtomicInteger> map = new ConcurrentHashMap<String, AtomicInteger>(); public void increment(String key) { AtomicInteger value = new AtomicInteger(0); AtomicInteger old = map.putIfAbsent(key, value); if (old != null) { value = old; } value.incrementAndGet(); // Increment the value atomically } public Integer getCount(String key) { AtomicInteger value = map.get(key); return (value == null) ? null : value.get(); } }
Risk Assessment
Non-atomic code can induce race conditions and affect program correctness.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON07- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class Vector, Class WeakReference
[[JavaThreads 04]] 8.2 "Synchronization and Collection Classes"
[[Goetz 06]] 4.4.1. Client-side Locking, 4.4.2. Composition and 5.2.1. ConcurrentHashMap
[[Lee 09]] "Map & Compound Operation"
CON06-J. Do not defer a thread that is holding a lock 11. Concurrency (CON) CON08-J. Do not invoke a superclass method or constructor from a synchronized region in the subclass